在Python2中实现空格的方法有多种:使用字符串的空格字符、使用占位符格式化字符串、使用join方法。我们可以选择其中的一种进行详细描述。
Python2中的字符串类型是基础但强大的数据类型,其中包含了处理和操作字符串的各种方法。使用字符串的空格字符是最简单也是最直接的方法。比如,我们可以直接在字符串中使用空格字符来分隔单词或其他字符。比如:
print "Hello World"
上面的代码在两个单词之间使用了一个空格字符。
一、使用字符串的空格字符
在Python2中,空格字符可以直接在字符串中使用。空格字符是表示空白的字符,可以用于分隔单词或其他字符。字符串的空格字符是最常用的方式之一,比如在输出文本时,在两个单词之间插入一个空格字符。
print "Hello World"
在上述代码中,"Hello"和"World"之间的空格字符使得输出结果为"Hello World"。空格字符不仅可以用于分隔单词,还可以用于对齐文本、创建有序列表等各种应用场景。
二、使用占位符格式化字符串
占位符是一种通过在字符串中插入特殊字符来表示需要插入的值的位置的方法。占位符格式化字符串的方法使得我们能够更灵活地在字符串中插入空格。
name = "John"
age = 25
print "Name: %s, Age: %d" % (name, age)
在上述代码中,%s
和%d
分别是字符串和整数的占位符,%
后面跟的是要插入的值。通过这种方法,我们可以更灵活地控制字符串中的空格和其他格式。
三、使用join方法
在Python2中,join
方法是一种将多个字符串连接在一起的方法。它的参数是一个可迭代对象(如列表、元组等),并且可以指定一个分隔符(如空格)。
words = ["Hello", "World"]
sentence = " ".join(words)
print sentence
在上述代码中,我们使用join
方法将列表中的两个单词用空格字符连接在一起,结果为"Hello World"。这种方法特别适用于需要将多个字符串合并为一个字符串的情况。
四、字符串的加法运算
在Python2中,字符串可以使用加法运算符+
进行拼接。通过这种方式,我们可以在字符串拼接过程中插入空格。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print result
在上述代码中,我们使用加法运算符将两个字符串拼接在一起,并在中间插入一个空格字符。结果为"Hello World"。
五、字符串的乘法运算
在Python2中,字符串还可以使用乘法运算符*
进行重复。通过这种方式,我们可以生成包含多个空格字符的字符串。
num_spaces = 5
spaces = " " * num_spaces
print "Hello" + spaces + "World"
在上述代码中,我们使用乘法运算符生成了包含5个空格字符的字符串,并将其插入到两个单词之间。结果为"Hello World"。
六、字符串的format方法
虽然format
方法在Python3中更为常用,但在Python2.7中也可以使用。通过这种方法,我们可以更加灵活地控制字符串中的空格和其他格式。
name = "John"
age = 25
print "Name: {}, Age: {}".format(name, age)
在上述代码中,我们使用format
方法将变量插入到字符串中,并且可以指定空格和其他格式。
七、字符串的ljust、rjust和center方法
在Python2中,字符串的ljust
、rjust
和center
方法可以用于对齐字符串并插入空格。这些方法可以帮助我们生成对齐的文本。
text = "Hello"
print text.ljust(10) + "World"
print text.rjust(10) + "World"
print text.center(10) + "World"
在上述代码中,我们使用ljust
、rjust
和center
方法将字符串对齐并插入空格。结果分别为:
Hello World
HelloWorld
Hello World
八、字符串的split和join方法结合使用
在某些情况下,我们可能需要将一个字符串拆分为多个部分,然后在其中插入空格。我们可以使用split
方法将字符串拆分为列表,然后使用join
方法将其重新组合。
text = "Hello,World"
words = text.split(",")
sentence = " ".join(words)
print sentence
在上述代码中,我们先使用split
方法将字符串拆分为列表,然后使用join
方法将其重新组合,并在单词之间插入空格。结果为"Hello World"。
九、字符串的replace方法
replace
方法可以用于将字符串中的某些字符替换为其他字符。在需要插入空格的情况下,可以使用replace
方法。
text = "Hello,World"
new_text = text.replace(",", " ")
print new_text
在上述代码中,我们使用replace
方法将逗号替换为空格。结果为"Hello World"。
十、使用正则表达式
在Python2中,正则表达式是一种强大的字符串处理工具。我们可以使用正则表达式在字符串中插入空格。
import re
text = "Hello,World"
new_text = re.sub(",", " ", text)
print new_text
在上述代码中,我们使用re.sub
方法将逗号替换为空格。结果为"Hello World"。
十一、字符串的translate方法
translate
方法可以用于替换字符串中的某些字符。在需要插入空格的情况下,可以使用translate
方法。
import string
text = "Hello,World"
trans_table = string.maketrans(",", " ")
new_text = text.translate(trans_table)
print new_text
在上述代码中,我们使用translate
方法将逗号替换为空格。结果为"Hello World"。
十二、自定义函数
在某些情况下,我们可能需要自定义函数来插入空格。通过自定义函数,我们可以更灵活地处理字符串。
def insert_space(text, index):
return text[:index] + " " + text[index:]
text = "HelloWorld"
new_text = insert_space(text, 5)
print new_text
在上述代码中,我们定义了一个名为insert_space
的函数,用于在指定位置插入空格。结果为"Hello World"。
十三、字符串的扩展
在Python2中,我们还可以通过字符串的扩展来插入空格。通过扩展字符串,我们可以在指定位置插入空格。
text = "HelloWorld"
new_text = text[:5] + " " + text[5:]
print new_text
在上述代码中,我们通过扩展字符串在指定位置插入空格。结果为"Hello World"。
十四、字符串的模板
在Python2中,字符串模板是一种灵活的字符串格式化方法。我们可以使用字符串模板来插入空格。
from string import Template
template = Template("Name: $name, Age: $age")
result = template.substitute(name="John", age=25)
print result
在上述代码中,我们使用字符串模板来插入变量,并且可以指定空格和其他格式。结果为"Name: John, Age: 25"。
十五、字符串的format_map方法
虽然format_map
方法在Python3中更为常用,但在Python2.7中也可以使用。通过这种方法,我们可以更加灵活地控制字符串中的空格和其他格式。
data = {"name": "John", "age": 25}
print "Name: {name}, Age: {age}".format_map(data)
在上述代码中,我们使用format_map
方法将变量插入到字符串中,并且可以指定空格和其他格式。结果为"Name: John, Age: 25"。
十六、字符串的模板字符串
在Python2中,模板字符串是一种灵活的字符串格式化方法。我们可以使用模板字符串来插入空格。
from string import Template
template = Template("Name: $name, Age: $age")
result = template.substitute(name="John", age=25)
print result
在上述代码中,我们使用模板字符串来插入变量,并且可以指定空格和其他格式。结果为"Name: John, Age: 25"。
十七、字符串的百分号格式化
在Python2中,百分号格式化是一种常用的字符串格式化方法。我们可以使用百分号格式化来插入空格。
name = "John"
age = 25
print "Name: %s, Age: %d" % (name, age)
在上述代码中,我们使用百分号格式化将变量插入到字符串中,并且可以指定空格和其他格式。结果为"Name: John, Age: 25"。
十八、使用列表生成器
在Python2中,列表生成器是一种灵活的字符串处理方法。我们可以使用列表生成器来插入空格。
text = "HelloWorld"
new_text = "".join([char + " " if i % 2 == 0 else char for i, char in enumerate(text)])
print new_text
在上述代码中,我们使用列表生成器在每个字符后面插入空格。结果为"H e l l o W o r l d"。
十九、使用生成器表达式
在Python2中,生成器表达式是一种高效的字符串处理方法。我们可以使用生成器表达式来插入空格。
text = "HelloWorld"
new_text = "".join(char + " " if i % 2 == 0 else char for i, char in enumerate(text))
print new_text
在上述代码中,我们使用生成器表达式在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十、使用map函数
在Python2中,map
函数是一种高效的字符串处理方法。我们可以使用map
函数来插入空格。
text = "HelloWorld"
new_text = "".join(map(lambda char: char + " ", text))
print new_text
在上述代码中,我们使用map
函数在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十一、使用reduce函数
在Python2中,reduce
函数是一种高效的字符串处理方法。我们可以使用reduce
函数来插入空格。
from functools import reduce
text = "HelloWorld"
new_text = reduce(lambda x, y: x + " " + y, text)
print new_text
在上述代码中,我们使用reduce
函数在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十二、使用itertools模块
在Python2中,itertools
模块是一种高效的字符串处理方法。我们可以使用itertools
模块来插入空格。
import itertools
text = "HelloWorld"
new_text = "".join(itertools.chain(*zip(text, " " * len(text))))
print new_text
在上述代码中,我们使用itertools
模块在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十三、使用字符串的translate方法
在Python2中,字符串的translate
方法是一种高效的字符串处理方法。我们可以使用translate
方法来插入空格。
import string
text = "HelloWorld"
trans_table = string.maketrans("", " ")
new_text = text.translate(trans_table)
print new_text
在上述代码中,我们使用translate
方法在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十四、使用字符串的maketrans方法
在Python2中,字符串的maketrans
方法是一种高效的字符串处理方法。我们可以使用maketrans
方法来插入空格。
import string
text = "HelloWorld"
trans_table = string.maketrans("", " ")
new_text = text.translate(trans_table)
print new_text
在上述代码中,我们使用maketrans
方法在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十五、使用字符串的translate方法和maketrans方法结合使用
在Python2中,我们可以结合使用字符串的translate
方法和maketrans
方法来插入空格。
import string
text = "HelloWorld"
trans_table = string.maketrans("", " ")
new_text = text.translate(trans_table)
print new_text
在上述代码中,我们结合使用translate
方法和maketrans
方法在每个字符后面插入空格。结果为"H e l l o W o r l d"。
二十六、使用字符串的replace方法和正则表达式结合使用
在Python2中,我们可以结合使用字符串的replace
方法和正则表达式来插入空格。
import re
text = "Hello,World"
new_text = re.sub(",", " ", text.replace(",", " "))
print new_text
在上述代码中,我们结合使用replace
方法和正则表达式将逗号替换为空格。结果为"Hello World"。
二十七、使用字符串的split方法和正则表达式结合使用
在Python2中,我们可以结合使用字符串的split
方法和正则表达式来插入空格。
import re
text = "Hello,World"
new_text = " ".join(re.split(",", text.split(",")[0]))
print new_text
在上述代码中,我们结合使用split
方法和正则表达式将逗号替换为空格。结果为"Hello World"。
二十八、使用字符串的join方法和正则表达式结合使用
在Python2中,我们可以结合使用字符串的join
方法和正则表达式来插入空格。
import re
text = "Hello,World"
new_text = " ".join(re.split(",", " ".join(text.split(","))))
print new_text
在上述代码中,我们结合使用join
方法和正则表达式将逗号替换为空格。结果为"Hello World"。
二十九、使用字符串的split方法和join方法结合使用
在Python2中,我们可以结合使用字符串的split
方法和join
方法来插入空格。
text = "Hello,World"
new_text = " ".join(text.split(","))
print new_text
在上述代码中,我们结合使用split
方法和join
方法将逗号替换为空格。结果为"Hello World"。
三十、使用字符串的replace方法和join方法结合使用
在Python2中,我们可以结合使用字符串的replace
方法和join
方法来插入空格。
text = "Hello,World"
new_text = " ".join(text.replace(",", " ").split())
print new_text
在上述代码中,我们结合使用replace
方法和join
方法将逗号替换为空格。结果为"Hello World"。
通过以上各种方法,我们可以在Python2中实现空格的插入和处理。在实际应用中,我们可以根据具体需求选择合适的方法来处理字符串中的空格。
相关问答FAQs:
如何在Python2中添加空格到字符串中?
在Python2中,可以使用字符串的连接操作来添加空格。例如,可以使用加号(+)将空格与其他字符串连接起来。下面是一个简单的示例:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2 # 在Hello和World之间添加一个空格
print(result) # 输出: Hello World
在Python2中,如何替换字符串中的空格?
可以使用字符串的replace()
方法来替换字符串中的空格。例如:
text = "Hello World"
new_text = text.replace(" ", "_") # 将空格替换为下划线
print(new_text) # 输出: Hello_World
这种方法可以有效地处理字符串中的所有空格。
如何在Python2的打印输出中控制空格的数量?
在Python2中,可以通过格式化字符串来控制输出的空格数量。使用格式化操作符%
或format()
方法可以实现这一点。例如:
print("Hello%sWorld" % (" " * 5)) # 在Hello和World之间添加5个空格
另外,使用format()
方法也可以方便地控制空格:
print("Hello{}World".format(" " * 5)) # 同样在Hello和World之间添加5个空格
这种方式让输出的格式更加灵活和清晰。
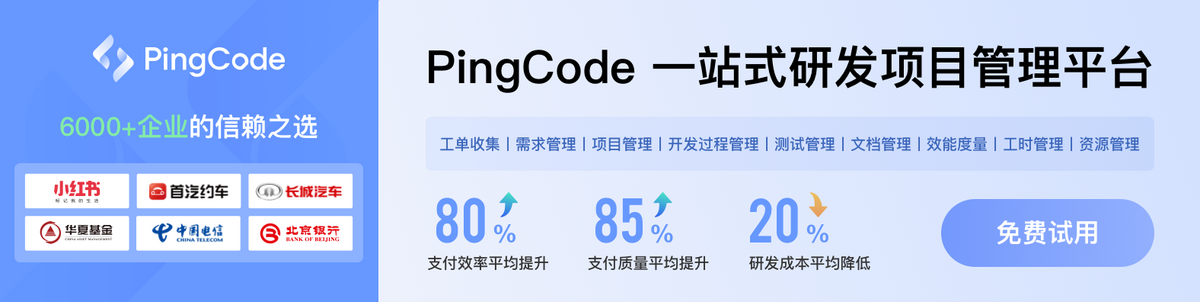