forEach在JavaScript中是一种数组迭代方法,它允许你对数组的每个元素执行一个函数。它不返回值、不能链式调用,以及不可在回调中中断循环。 forEach方法接收一个回调函数作为参数,该回调函数又可接收当前元素、当前元素的索引及整个数组三个参数。forEach对于执行数组元素的侧重效率和简洁的遍历操作非常有用。
以一个简单的例子来展开描述,比如有一个数组[1, 2, 3]
,并想为每个元素打印出值。可以这样使用forEach:
const array = [1, 2, 3];
array.forEach(function(element) {
console.log(element);
});
这段代码会依次输出1、2、3。forEach方法是处理数组元素的强大工具,可用于替代传统的for循环,让代码更简洁、更易读。
一、FOREACH 基础使用
forEach循环的基础用法是遍历数组的每一个元素并对其执行回调函数。你可以使用匿名函数或箭头函数作为回调函数。
匿名函数作为回调
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(function(item, index, array) {
console.log(item, index);
});
在上述代码中,匿名函数接收每个水果名称、它的索引和数组本身作为参数,并将名称和索引打印出来。
箭头函数作为回调
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number, index) => {
console.log(`Index ${index}: ${number}`);
});
此处使用了ES6引入的箭头函数,它提供了一种更简洁的函数书写方式,作用相同。
二、FOREACH 与 MAP、FILTER、REDUCE 的比较
虽然forEach是处理数组的基本方法,但在处理数组时经常需要根据元素生成新的数组或值,在这些场景中可以使用map、filter和reduce。
和map的区别
forEach无返回值,而map返回新数组。如果想获取一个基于原数组每个元素转换后的新数组,应使用map。
const squares = numbers.map(number => number * number);
console.log(squares); // 输出: [1, 4, 9, 16, 25]
和filter的区别
filter用于根据条件筛选数组,创建一个包含通过测试的所有元素的新数组。
const even = numbers.filter(number => number % 2 === 0);
console.log(even); // 输出: [2, 4]
和reduce的区别
reduce可以累积数组中的值,生成单一的结果。
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // 输出: 15
三、FOREACH 中的异步操作
在forEach循环中执行异步操作可能会出现问题,因为forEach不会等待异步操作的完成。如果需要使用异步函数处理数组,应考虑使用for-of循环或Promise.all。
const urls = ['url1', 'url2', 'url3'];
// 错误的异步使用
urls.forEach(async (url) => {
const response = awAIt fetch(url); // fetch是一个异步操作
const content = await response.text();
console.log(content);
});
// 正确的异步处理方式 - 使用Promise.all
Promise.all(urls.map(url => fetch(url).then(resp => resp.text()))).then(contents => {
contents.forEach(content => {
console.log(content);
});
});
四、FOREACH 使用常见误区
在使用forEach时,有几个常见的误区需要避免。
不能使用break或continue
在forEach循环中不能使用break或continue语句来中断或跳过迭代。
const myArray = [1, 2, 3, 4, 5];
// 不能这样做,会抛出错误
myArray.forEach(number => {
if (number > 3) break; // SyntaxError: Illegal break statement
console.log(number);
});
forEach不会直接修改原始数组
虽然可以在forEach的回调中修改原始数组的元素,它本身不会直接修改数组。
const myArray = [1, 2, 3];
myArray.forEach((number, index) => {
myArray[index] = number * 2;
});
console.log(myArray); // 输出: [2, 4, 6]
尽管在forEach中更改了数组,但这是由于显式的赋值操作,而不是forEach本身的功能。
五、FOREACH 在现代JavaScript开发中的应用场景
在现代JavaScript开发中,forEach用于替代传统的for循环,用于数组的迭代。
面向对象的方法遍历
当你在处理属于某个对象方法的数组时,forEach提供了一种将上下文传递给回调函数的便捷方式。
class Printer {
constructor() {
this.docs = ['doc1', 'doc2', 'doc3'];
}
printDocs() {
this.docs.forEach(function(doc) {
console.log(doc);
}, this);
}
}
const printer = new Printer();
printer.printDocs();
在上面的例子中,this
关键字被用作forEach的第二个参数,确保回调函数在正确的上下文中执行。
联动性操作
处理DOM元素的列表时,forEach被广泛使用,如给多个DOM元素添加事件监听或者修改样式等。
const buttons = document.querySelectorAll('button');
buttons.forEach(button => {
button.addEventListener('click', () => {
console.log('Button clicked!');
});
});
在这个例子中,给页面上的所有按钮添加了点击事件监听。
总结,forEach是JavaScript中对数组进行迭代的重要方法,适合用于数组的遍历和操作元素,但在处理返回新数组、筛选或累积值等需求时,有其他更适合的迭代方法可用。在使用forEach时,特别需要注意它不能中断循环,且不适合异步操作的直接使用。
相关问答FAQs:
1. JavaScript中如何使用forEach方法?
在JavaScript中,forEach是数组对象的一个方法,用于遍历数组并对其中的每个元素执行一次指定的回调函数。下面是使用forEach方法的示例代码:
const array = [1, 2, 3, 4, 5];
array.forEach((element) => {
console.log(element);
});
上述代码会依次输出数组中的每个元素。通过传入一个回调函数,我们可以在每次遍历数组时执行特定的操作。
2. 如何在forEach循环中使用索引值?
在使用forEach方法时,我们可以通过添加第二个可选参数来获取当前元素的索引值。下面是一个例子:
const array = [1, 2, 3, 4, 5];
array.forEach((element, index) => {
console.log(`元素 ${element} 的索引值为 ${index}`);
});
通过此方法,我们可以在循环中同时访问元素和其对应的索引值。
3. forEach循环中如何使用break或continue语句?
forEach方法是一个纯粹的迭代方法,不能直接使用break或continue语句来提前结束循环或跳过某个元素。但是,我们可以通过抛出异常来模拟类似的效果。
下面是一个使用try和catch语句来实现类似break和continue的示例:
const array = [1, 2, 3, 4, 5];
try {
array.forEach((element) => {
if (element === 3) {
throw "跳过当前元素";
}
// 执行某些操作
if (element === 5) {
throw "结束循环";
}
});
} catch (error) {
if (error !== "跳过当前元素" && error !== "结束循环") {
throw error;
}
}
这种方式虽然不太直观,但在forEach方法中实现跳出循环还是可以做到的。
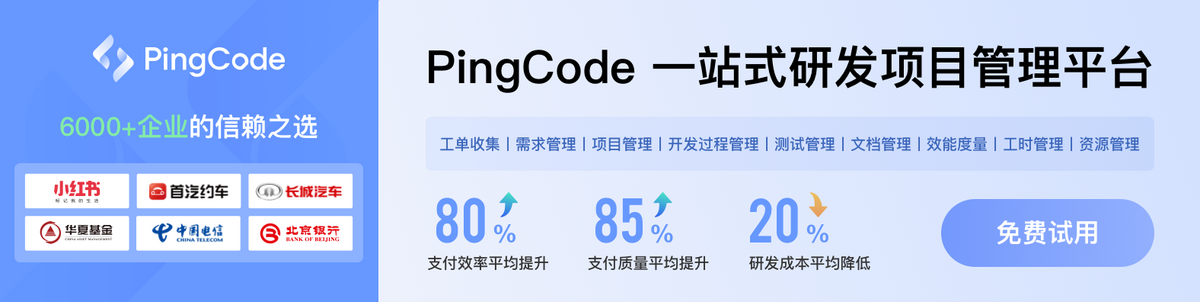