要在Python中实现定时发送邮件,可以使用调度库(如schedule、APScheduler)、结合SMTP库(如smtplib)和电子邮件构建库(如email.mime),通过编写脚本实现自动化。我们将详细介绍如何通过这些工具实现这一功能。
一、定时任务调度与发送邮件
在Python中,实现定时任务调度的常用方法包括使用schedule库和APScheduler库。这些库提供了简单的接口,可以轻松地安排和管理定时任务。
- 使用schedule库
Schedule库是一个轻量级的任务调度库,适用于简单的定时任务。首先,需要安装schedule库:
pip install schedule
使用schedule库的基本步骤如下:
- 导入必要的库:包括schedule和time。
- 定义一个函数,用于执行定时任务。此函数将负责构建和发送邮件。
- 使用schedule.every()方法设置任务的执行间隔,例如每小时、每天等。
- 使用schedule.run_pending()在循环中检查并执行任务。
import schedule
import time
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart("alternative")
message["Subject"] = "定时邮件"
message["From"] = sender_email
message["To"] = receiver_email
text = """\
你好,
这是一封定时发送的邮件。
"""
part = MIMEText(text, "plain")
message.attach(part)
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
schedule.every().day.at("10:00").do(send_email)
while True:
schedule.run_pending()
time.sleep(1)
- 使用APScheduler库
APScheduler是一个功能更强大的调度库,适合需要更复杂调度需求的场景。首先,需要安装APScheduler库:
pip install apscheduler
APScheduler支持多种调度器,如BlockingScheduler、BackgroundScheduler等。以下是使用APScheduler实现定时邮件发送的示例:
from apscheduler.schedulers.blocking import BlockingScheduler
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart("alternative")
message["Subject"] = "定时邮件"
message["From"] = sender_email
message["To"] = receiver_email
text = """\
你好,
这是一封定时发送的邮件。
"""
part = MIMEText(text, "plain")
message.attach(part)
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
scheduler = BlockingScheduler()
scheduler.add_job(send_email, 'cron', hour=10, minute=0)
scheduler.start()
二、构建邮件内容
在发送邮件之前,需要构建邮件的内容。Python的email库提供了多种方式来构建邮件内容,包括纯文本邮件、HTML格式邮件以及带附件的邮件。
- 构建纯文本邮件
上文中的示例代码已经展示了如何使用MIMEText构建纯文本邮件。需要注意的是,MIMEText的第二个参数指定了文本的类型,可以是"plain"(纯文本)或"html"(HTML格式)。
- 构建HTML格式邮件
为了提高邮件的表现力,可以使用HTML格式的邮件。修改邮件内容为HTML格式,只需将MIMEText的第二个参数改为"html"。
html = """\
<html>
<body>
<p>你好,<br>
这是一封定时发送的邮件。<br>
<a href="http://www.example.com">点击这里</a>查看更多信息。
</p>
</body>
</html>
"""
part = MIMEText(html, "html")
message.attach(part)
- 添加附件
为了发送带附件的邮件,可以使用MIMEBase来添加附件。以下是一个示例:
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart()
message["Subject"] = "定时邮件"
message["From"] = sender_email
message["To"] = receiver_email
text = "你好,\n这是一封带附件的定时邮件。"
message.attach(MIMEText(text, "plain"))
filename = "document.pdf"
with open(filename, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header(
"Content-Disposition",
f"attachment; filename= {filename}",
)
message.attach(part)
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
send_email_with_attachment()
三、SMTP服务器设置
在使用smtplib发送邮件时,需要连接到SMTP服务器。大多数电子邮件服务提供商都提供SMTP服务器信息。以下是一些常见的SMTP服务器配置:
- Gmail: smtp.gmail.com, 端口465(SSL)
- Outlook: smtp-mail.outlook.com, 端口587(TLS)
- Yahoo Mail: smtp.mail.yahoo.com, 端口465(SSL)
使用smtplib.SMTP_SSL()方法连接到SSL端口,或者使用smtplib.SMTP()并调用starttls()方法连接到TLS端口。
四、处理发送失败的情况
在发送邮件时,可能会遇到各种错误,如网络问题、身份验证失败等。为了提高程序的鲁棒性,可以在发送邮件时捕获异常,并进行相应的处理。
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("邮件发送成功")
except smtplib.SMTPAuthenticationError:
print("身份验证失败,请检查用户名和密码")
except smtplib.SMTPConnectError:
print("无法连接到SMTP服务器")
except smtplib.SMTPException as e:
print(f"邮件发送失败: {e}")
五、总结
通过结合Python的schedule或APScheduler库、smtplib库以及email.mime库,可以实现定时发送邮件的功能。掌握这些工具的使用方法后,可以根据自己的需求进行定制和扩展。无论是简单的定时任务,还是复杂的调度需求,Python都能提供强大的支持,帮助我们实现高效的自动化工作流程。
相关问答FAQs:
如何使用Python定时发送邮件?
Python可以通过结合定时任务和邮件发送库来实现定时发送邮件。常用的邮件发送库如smtplib
可以与schedule
库配合使用。你可以编写一个脚本,设置特定的时间来发送邮件,而schedule
库可以帮助你安排这些任务。通过设置合适的时间间隔和发送频率,你可以轻松实现定时邮件的功能。
在Python中,发送邮件需要哪些库和工具?
发送邮件的主要库是smtplib
,它允许你通过SMTP协议与邮件服务器进行通信。此外,email
库可以帮助你构建邮件内容,如主题、发件人和收件人等信息。在定时发送的场景下,schedule
库或time
模块也常被用来设置定时任务。确保在使用这些库时,你的环境中已经安装了相关依赖。
如何处理邮件发送中的异常情况?
在定时发送邮件的过程中,可能会遇到网络问题、SMTP服务器故障或认证失败等异常情况。建议在代码中加入异常处理机制,例如使用try-except
块来捕获异常,并记录错误信息。这样可以确保即使出现问题,程序也能正常运行,并在后续重新尝试发送邮件。确保你还可以通过日志记录邮件的发送状态,以便后续排查和管理。
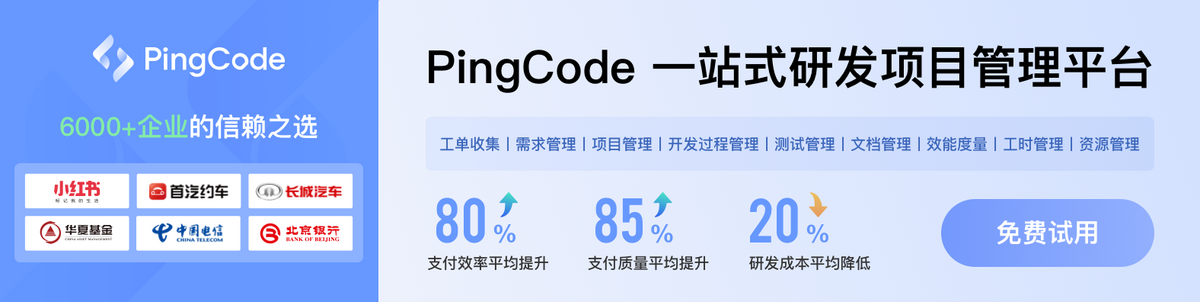