在Python中绘制爱心图形,可以通过使用数学公式、绘图库如Matplotlib和Turtle、ASCII字符等方法实现。
下面我将详细介绍使用Matplotlib绘制爱心图形的方法。
一、使用MATPLOTLIB绘制爱心图形
Matplotlib是Python中一个强大的绘图库,支持多种图形的绘制。我们可以通过数学方程来绘制爱心图形。
-
数学方程介绍
爱心形状可以通过参数方程来定义。经典的爱心形状可以用以下参数方程表示:
[
x = 16 \sin^3(t)
]
[
y = 13 \cos(t) – 5 \cos(2t) – 2 \cos(3t) – \cos(4t)
]
其中,( t ) 是从 0 到 ( 2\pi ) 的参数。
-
使用Matplotlib绘制
首先,我们需要安装Matplotlib库。如果尚未安装,可以通过以下命令进行安装:
pip install matplotlib
然后,可以使用以下代码绘制爱心图形:
import numpy as np
import matplotlib.pyplot as plt
生成t的值
t = np.linspace(0, 2 * np.pi, 1000)
计算x和y的值
x = 16 * np.sin(t) 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
创建图形
plt.figure(figsize=(8, 6))
plt.plot(x, y, color='red')
设置图形属性
plt.title("Heart Shape")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.axis('equal') # 保证图形比例
plt.show()
这个代码通过numpy生成参数t的值,然后计算出x和y的值,并使用Matplotlib绘制出爱心的形状。
二、使用TURTLE绘制爱心图形
Turtle是Python内置的一个简单绘图库,非常适合用来创建简单的图形和动画。
-
安装和使用Turtle
Turtle库不需要额外安装,因为它是Python标准库的一部分。可以直接使用。
-
绘制爱心
使用Turtle绘制爱心的代码如下:
import turtle
设置窗口
screen = turtle.Screen()
screen.setup(width=600, height=600)
screen.title("Heart Shape with Turtle")
创建Turtle对象
pen = turtle.Turtle()
设置笔的速度和颜色
pen.speed(3)
pen.color("red")
开始绘制爱心
pen.begin_fill()
pen.left(140)
pen.forward(224)
绘制爱心的左半部分
pen.circle(-112, 200)
绘制爱心的右半部分
pen.left(120)
pen.circle(-112, 200)
pen.forward(224)
填充颜色
pen.end_fill()
隐藏Turtle
pen.hideturtle()
保持窗口打开
screen.mainloop()
这段代码使用Turtle库创建了一个窗口,并绘制了一个红色的爱心形状。
三、使用ASCII字符绘制爱心
如果您希望在控制台中显示一个简单的爱心,可以使用ASCII字符。
-
ASCII字符绘制
通过组合字符,可以创建一个简单的爱心形状。以下是一个例子:
heart = [
" <strong>* </strong>* ",
" <strong></strong>* <strong></strong>* ",
"<strong></strong><strong></strong><strong></strong>*",
" <strong></strong><strong></strong>* ",
" <strong></strong><strong></strong>* ",
" <strong></strong>* ",
" <strong></strong>* ",
" * ",
" * "
]
for line in heart:
print(line)
这个代码使用简单的字符模式在控制台中打印出一个爱心形状。
四、总结
在Python中绘制爱心图形有多种方法,具体选择哪种方法可以根据需求和应用场景决定。Matplotlib适合需要精确控制图形属性和样式的情况,Turtle适合创建简单动画和图形,而ASCII字符适合在文本环境中显示简单形状。通过这些方法,您可以轻松在Python中创建各种风格的爱心图形。
相关问答FAQs:
如何用Python绘制爱心图形?
要在Python中绘制爱心图形,可以使用一些图形库,比如turtle
或matplotlib
。使用turtle
库,你可以通过绘制曲线和线条来形成爱心的形状。示例代码如下:
import turtle
t = turtle.Turtle()
t.fillcolor("red")
t.begin_fill()
t.left(140)
t.forward(224)
t.circle(-112, 200)
t.left(120)
t.circle(-112, 200)
t.forward(224)
t.end_fill()
turtle.done()
运行这段代码后,你会看到一个红色的爱心图形。
有哪些Python库可以用于绘制爱心形状?
除了turtle
,还有其他一些库可以用来绘制爱心形状。例如,使用matplotlib
库,你可以创建更复杂的图形。在matplotlib
中,可以通过绘制函数来形成爱心形状。示例代码如下:
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
plt.fill(x, y, color='red')
plt.title("Heart Shape")
plt.axis('equal')
plt.show()
这段代码将会生成一个填充红色的爱心图案。
如何在Python中给爱心图形添加动画效果?
如果你想为爱心图形添加动画效果,可以使用turtle
库的动画功能,或使用matplotlib
的动态更新功能。对于turtle
,你可以通过循环绘制爱心并调整颜色或大小来创建简单的动画。对于matplotlib
,可以使用FuncAnimation
来实现动态效果。示例代码如下:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
t = np.linspace(0, 2 * np.pi, 1000)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
line, = ax.fill(x, y, color='red')
def update(frame):
line.set_alpha(0.5 + 0.5 * np.sin(frame / 10))
return line,
ani = FuncAnimation(fig, update, frames=np.arange(0, 100), blit=True)
plt.axis('equal')
plt.show()
这段代码将会生成一个具有透明度变化的爱心动画。
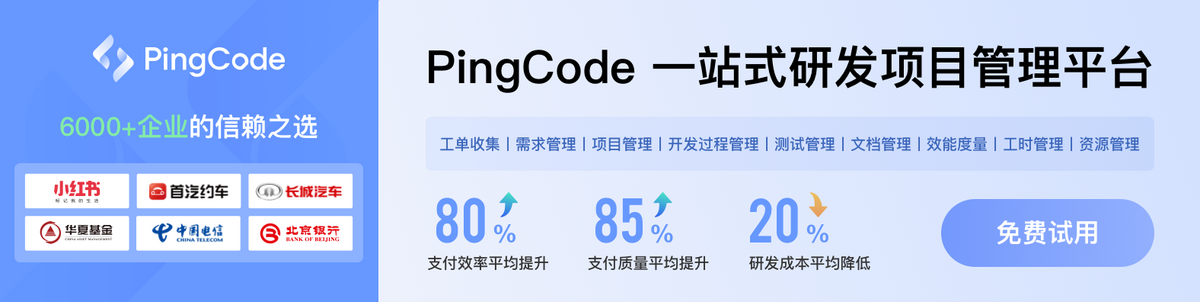