要让Python下载exe文件,可以使用requests
库进行HTTP请求、使用urllib
库处理下载链接、确保目标网址的合法性和安全性。我们将详细介绍如何使用这些方法来下载exe文件,并讨论相关的安全和效率考虑。
一、使用requests
库下载exe文件
requests
库是Python中用于发送HTTP请求的流行库。它简单易用,非常适合下载文件。
-
安装和导入requests库
在开始之前,确保已经安装了
requests
库。如果没有安装,可以通过以下命令进行安装:pip install requests
然后在Python脚本中导入该库:
import requests
-
发送HTTP请求并下载文件
使用
requests.get()
方法发送HTTP请求,并使用shutil
库将内容写入文件中:import requests
import shutil
url = 'http://example.com/file.exe'
response = requests.get(url, stream=True)
with open('downloaded_file.exe', 'wb') as file:
shutil.copyfileobj(response.raw, file)
在此代码中,
stream=True
允许我们逐块读取数据,这对于下载大文件非常有用。 -
处理错误和异常
始终检查HTTP响应代码,并处理可能的异常:
try:
response = requests.get(url, stream=True)
response.raise_for_status() # 检查请求是否成功
with open('downloaded_file.exe', 'wb') as file:
shutil.copyfileobj(response.raw, file)
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"An error occurred: {err}")
通过这种方式,我们可以确保即使在下载过程中发生错误,也可以得到适当的处理。
二、使用urllib
库下载exe文件
urllib
库是Python标准库的一部分,可以用于处理URL和下载文件。
-
使用
urllib
下载文件urllib
库提供了一个简单的接口来下载文件:import urllib.request
url = 'http://example.com/file.exe'
urllib.request.urlretrieve(url, 'downloaded_file.exe')
-
处理下载进度
如果需要显示下载进度,可以自定义一个回调函数:
import urllib.request
def download_progress(block_num, block_size, total_size):
downloaded = block_num * block_size
percentage = downloaded / total_size * 100
print(f"Downloaded: {percentage:.2f}%")
url = 'http://example.com/file.exe'
urllib.request.urlretrieve(url, 'downloaded_file.exe', reporthook=download_progress)
这种方法可以提供用户友好的反馈。
三、确保目标网址的合法性和安全性
在下载exe文件时,确保从合法和安全的来源下载,以防止下载恶意软件。
-
验证URL的合法性
确保URL来自可信任的来源,并使用HTTPS协议以确保数据的完整性和安全性。
-
使用防病毒软件扫描下载的文件
在执行下载的exe文件之前,使用防病毒软件进行扫描,以确保文件没有被篡改。
-
检查文件的哈希值
如果提供哈希值,请在下载后验证文件的完整性:
import hashlib
def check_file_hash(file_path, expected_hash):
with open(file_path, 'rb') as file:
file_hash = hashlib.sha256(file.read()).hexdigest()
return file_hash == expected_hash
使用示例
expected_hash = 'expected_hash_value'
if check_file_hash('downloaded_file.exe', expected_hash):
print("File is intact.")
else:
print("File has been altered.")
四、下载效率与性能优化
在下载大文件时,效率和性能是重要的考虑因素。
-
使用多线程下载
对于大型文件,使用多线程可以显著提高下载速度。可以使用
concurrent.futures
库来实现:import requests
from concurrent.futures import ThreadPoolExecutor
def download_chunk(url, start, end, file_name):
headers = {'Range': f'bytes={start}-{end}'}
response = requests.get(url, headers=headers, stream=True)
with open(file_name, 'r+b') as file:
file.seek(start)
file.write(response.content)
def download_file(url, file_name, num_threads=4):
response = requests.head(url)
file_size = int(response.headers['Content-Length'])
with open(file_name, 'wb') as file:
file.truncate(file_size)
chunk_size = file_size // num_threads
with ThreadPoolExecutor(max_workers=num_threads) as executor:
futures = []
for i in range(num_threads):
start = i * chunk_size
end = file_size if i == num_threads - 1 else (i + 1) * chunk_size - 1
futures.append(executor.submit(download_chunk, url, start, end, file_name))
for future in futures:
future.result()
使用示例
download_file('http://example.com/largefile.exe', 'largefile.exe')
此方法将文件分成多个部分,并使用多个线程同时下载。
-
使用断点续传
如果下载中断,可以使用HTTP的
Range
头来实现断点续传:def download_with_resume(url, file_name):
headers = {}
if os.path.exists(file_name):
headers['Range'] = f"bytes={os.path.getsize(file_name)}-"
response = requests.get(url, headers=headers, stream=True)
with open(file_name, 'ab') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
使用示例
download_with_resume('http://example.com/largefile.exe', 'largefile.exe')
这种方式可以在网络连接不稳定的情况下提升下载的可靠性。
五、总结与注意事项
下载exe文件时,确保从可信任的来源获取,并采取适当的安全措施,如验证文件的哈希值和使用防病毒软件扫描文件。此外,使用多线程和断点续传技术可以提高下载效率和可靠性。通过合理使用Python的requests
和urllib
库,可以实现高效、安全的文件下载。
相关问答FAQs:
如何使用Python下载exe文件?
使用Python下载exe文件可以通过多种方法实现,最常用的方法是使用内置的requests
库。可以通过以下步骤完成下载:
- 安装
requests
库(如果尚未安装),可以使用命令pip install requests
。 - 使用
requests.get()
方法获取exe文件的URL,接着将其写入本地文件中。以下是一个简单的示例代码:import requests url = 'http://example.com/file.exe' # 替换为实际的exe文件链接 response = requests.get(url) with open('downloaded_file.exe', 'wb') as file: file.write(response.content)
- 运行代码后,exe文件将被下载到当前工作目录中。
在下载exe文件时需要注意哪些事项?
下载exe文件时,应确保来源的安全性。建议只从可信的网站下载文件,避免因下载恶意软件而导致安全风险。此外,检查文件大小和MD5值可以帮助确认文件的完整性和真实性。
如何处理下载过程中的异常情况?
在下载exe文件时,可能会遇到网络问题或文件不存在等异常情况。可以使用try-except
语句处理这些异常。例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
except requests.exceptions.RequestException as e:
print(f"下载失败: {e}")
这样可以确保程序在遇到问题时不会崩溃,并能给出相应的错误提示。
下载的exe文件如何验证其完整性?
下载完成后,可以通过计算文件的哈希值进行验证。常用的哈希算法有MD5和SHA256。可以使用Python的hashlib
库来实现这一功能。以下是一个简单的示例:
import hashlib
def get_md5(file_path):
hash_md5 = hashlib.md5()
with open(file_path, "rb") as f:
for chunk in iter(lambda: f.read(4096), b""):
hash_md5.update(chunk)
return hash_md5.hexdigest()
print(get_md5('downloaded_file.exe')) # 输出下载文件的MD5值
这可以帮助确保下载的exe文件与原始文件一致。
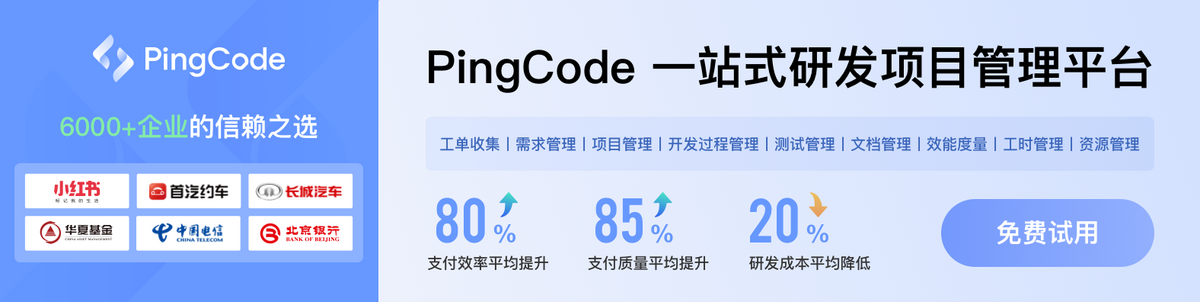