用Python写XML文件可以通过使用内置的xml.etree.ElementTree模块、lxml库、以及第三方库如xml.dom.minidom。最常用的方法是使用xml.etree.ElementTree模块,因为它简单易用、性能良好。下面将详细介绍如何使用xml.etree.ElementTree来生成XML文件。
1. 使用ElementTree模块
ElementTree模块是Python标准库中的一个模块,专门用于处理XML数据。它提供了一个简单的API来创建、解析和修改XML文档。以下是使用ElementTree模块创建XML文件的步骤:
- 创建根元素:XML文档的根元素是所有其他元素的父元素。使用
ElementTree.Element()
方法可以创建根元素。 - 创建子元素:使用
ElementTree.SubElement()
方法可以为根元素或其他子元素创建子元素。 - 为元素添加属性和文本:可以使用
set()
方法为元素添加属性,使用text
属性为元素添加文本。 - 将XML树写入文件:使用
ElementTree.ElementTree()
创建XML树,然后使用write()
方法将其写入文件。
import xml.etree.ElementTree as ET
创建根元素
root = ET.Element("data")
创建子元素
item1 = ET.SubElement(root, "item")
item1.set("name", "item1")
item1.text = "This is item 1"
item2 = ET.SubElement(root, "item")
item2.set("name", "item2")
item2.text = "This is item 2"
创建XML树并写入文件
tree = ET.ElementTree(root)
tree.write("output.xml", encoding='utf-8', xml_declaration=True)
一、INTRODUCTION TO XML AND PYTHON
XML (eXtensible Markup Language) is a markup language designed to store and transport data. Its structure is similar to HTML, consisting of tags and attributes, but it's more flexible and allows users to define their own custom tags. XML is widely used in web services, configuration files, and data interchange between applications.
Python, a versatile and powerful programming language, provides several libraries to work with XML data. These libraries allow developers to parse, create, and manipulate XML files efficiently. Understanding the basics of XML and Python's capabilities in handling XML is crucial for developers working in domains such as web development, data science, and software engineering.
- XML Structure and Elements
XML documents are composed of elements, which can contain text, attributes, and other elements. Each element is defined by a start tag and an end tag. Attributes provide additional information about elements and are defined within the start tag. XML documents must have a single root element that contains all other elements.
Example of a simple XML document:
<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend!</body>
</note>
- Importance of XML in Data Exchange
XML plays a vital role in data exchange across different systems and platforms. Its platform-independent nature makes it an ideal choice for web services and APIs. XML files are human-readable, making them easy to understand and debug. Additionally, XML can represent complex data structures, which is essential for applications requiring detailed data representation.
- Python Libraries for XML Processing
Python offers several libraries for XML processing, including:
xml.etree.ElementTree
: A lightweight and efficient library for parsing and creating XML documents.xml.dom.minidom
: A minimal implementation of the Document Object Model interface, useful for parsing and creating XML documents.lxml
: A powerful library for XML and HTML processing, providing advanced features and better performance.
Each library has its strengths and is suitable for different use cases. Choosing the right library depends on the complexity of the XML data and the specific requirements of the project.
二、WORKING WITH ELEMENTTREE
ElementTree is a popular Python library for XML processing due to its simplicity and efficiency. It provides a straightforward API for creating, parsing, and manipulating XML documents. Here, we'll explore how to use ElementTree to work with XML data.
- Creating XML Documents with ElementTree
ElementTree allows developers to create XML documents programmatically. The process involves creating elements, setting attributes, adding text, and organizing elements hierarchically.
Example of creating an XML document:
import xml.etree.ElementTree as ET
Create the root element
root = ET.Element("library")
Create a book element
book = ET.SubElement(root, "book")
book.set("id", "1")
Add title, author, and year elements
title = ET.SubElement(book, "title")
title.text = "Python Programming"
author = ET.SubElement(book, "author")
author.text = "John Doe"
year = ET.SubElement(book, "year")
year.text = "2023"
Create an ElementTree object and write to a file
tree = ET.ElementTree(root)
tree.write("library.xml", encoding='utf-8', xml_declaration=True)
- Parsing XML Documents with ElementTree
ElementTree can also parse existing XML documents, allowing developers to extract and manipulate data. The parse()
method reads an XML file and returns an ElementTree object. The find()
and findall()
methods are used to locate elements within the XML tree.
Example of parsing an XML document:
import xml.etree.ElementTree as ET
Parse the XML file
tree = ET.parse('library.xml')
root = tree.getroot()
Find and print book titles
for book in root.findall('book'):
title = book.find('title').text
print(f"Title: {title}")
- Modifying XML Documents with ElementTree
ElementTree enables developers to modify XML documents by adding, removing, or updating elements and attributes. Changes can be saved back to the original file or a new file.
Example of modifying an XML document:
import xml.etree.ElementTree as ET
Parse the XML file
tree = ET.parse('library.xml')
root = tree.getroot()
Add a new book element
new_book = ET.SubElement(root, "book")
new_book.set("id", "2")
title = ET.SubElement(new_book, "title")
title.text = "Advanced Python"
author = ET.SubElement(new_book, "author")
author.text = "Jane Smith"
year = ET.SubElement(new_book, "year")
year.text = "2024"
Write the modified XML to a file
tree.write("library_modified.xml", encoding='utf-8', xml_declaration=True)
三、ADVANCED XML PROCESSING WITH LXML
While ElementTree is sufficient for basic XML processing, the lxml library offers advanced features and better performance for complex XML documents. It provides a more comprehensive API and supports XPath and XSLT, making it a powerful tool for XML manipulation.
- Introduction to lxml
lxml is a third-party library that combines the power of libxml2 and libxslt libraries, providing a fast and feature-rich XML processing library for Python. It supports both XML and HTML processing and offers a wide range of functionalities, including XPath, XSLT, and schema validation.
- Creating XML Documents with lxml
Creating XML documents with lxml is similar to ElementTree, but with additional features and better performance. The Element
and SubElement
functions are used to create elements, while the tostring()
method generates the XML string.
Example of creating an XML document with lxml:
from lxml import etree
Create the root element
root = etree.Element("library")
Create a book element
book = etree.SubElement(root, "book", id="1")
Add title, author, and year elements
etree.SubElement(book, "title").text = "Python Programming"
etree.SubElement(book, "author").text = "John Doe"
etree.SubElement(book, "year").text = "2023"
Convert the XML tree to a string
xml_string = etree.tostring(root, pretty_print=True, xml_declaration=True, encoding='UTF-8')
Write the XML string to a file
with open('library_lxml.xml', 'wb') as f:
f.write(xml_string)
- Parsing and Modifying XML Documents with lxml
lxml provides robust methods for parsing and modifying XML documents. The parse()
method reads an XML file, and XPath expressions can be used to locate elements within the XML tree.
Example of parsing and modifying an XML document with lxml:
from lxml import etree
Parse the XML file
tree = etree.parse('library_lxml.xml')
root = tree.getroot()
Find and print book titles using XPath
for book in root.xpath('//book'):
title = book.find('title').text
print(f"Title: {title}")
Add a new book element
new_book = etree.SubElement(root, "book", id="2")
etree.SubElement(new_book, "title").text = "Advanced Python"
etree.SubElement(new_book, "author").text = "Jane Smith"
etree.SubElement(new_book, "year").text = "2024"
Write the modified XML to a file
tree.write("library_lxml_modified.xml", pretty_print=True, xml_declaration=True, encoding='UTF-8')
四、USING XML.DOME.MINIDOM FOR XML FORMATTING
xml.dom.minidom is a minimal implementation of the Document Object Model interface, useful for parsing and creating XML documents. It is part of Python's standard library and offers a more verbose API compared to ElementTree. However, it is beneficial for pretty-printing XML documents.
- Introduction to xml.dom.minidom
xml.dom.minidom provides a simple and lightweight way to parse and create XML documents. It represents the document as a tree structure with nodes, similar to the DOM model used in web development. This library is particularly useful for developers who need to manipulate XML documents in a more detailed manner.
- Creating and Formatting XML Documents
While creating XML documents with xml.dom.minidom can be more verbose, it allows for precise control over the document structure. The Document
class is used to create a new XML document, and elements are created using the createElement()
method.
Example of creating and formatting an XML document:
from xml.dom import minidom
Create a new XML document
doc = minidom.Document()
Create the root element
root = doc.createElement('library')
doc.appendChild(root)
Create a book element
book = doc.createElement('book')
book.setAttribute('id', '1')
root.appendChild(book)
Add title, author, and year elements
title = doc.createElement('title')
title.appendChild(doc.createTextNode('Python Programming'))
book.appendChild(title)
author = doc.createElement('author')
author.appendChild(doc.createTextNode('John Doe'))
book.appendChild(author)
year = doc.createElement('year')
year.appendChild(doc.createTextNode('2023'))
book.appendChild(year)
Pretty-print the XML document
xml_str = doc.toprettyxml(indent=' ')
Write the XML string to a file
with open('library_minidom.xml', 'w') as f:
f.write(xml_str)
- Parsing and Modifying XML Documents
xml.dom.minidom provides methods to parse and modify XML documents. The parse()
method reads an XML file, and elements can be accessed and modified using the document tree structure.
Example of parsing and modifying an XML document:
from xml.dom import minidom
Parse the XML file
doc = minidom.parse('library_minidom.xml')
Get the root element
root = doc.documentElement
Find and print book titles
books = root.getElementsByTagName('book')
for book in books:
title = book.getElementsByTagName('title')[0].firstChild.data
print(f"Title: {title}")
Add a new book element
new_book = doc.createElement('book')
new_book.setAttribute('id', '2')
title = doc.createElement('title')
title.appendChild(doc.createTextNode('Advanced Python'))
new_book.appendChild(title)
author = doc.createElement('author')
author.appendChild(doc.createTextNode('Jane Smith'))
new_book.appendChild(author)
year = doc.createElement('year')
year.appendChild(doc.createTextNode('2024'))
new_book.appendChild(year)
root.appendChild(new_book)
Pretty-print the modified XML document
xml_str = doc.toprettyxml(indent=' ')
Write the modified XML string to a file
with open('library_minidom_modified.xml', 'w') as f:
f.write(xml_str)
五、BEST PRACTICES FOR XML PROCESSING IN PYTHON
When working with XML in Python, following best practices ensures efficient and maintainable code. Here are some tips and guidelines for effective XML processing:
- Choose the Right Library
Selecting the appropriate library for XML processing depends on the complexity of the XML data and the specific requirements of the project. Use ElementTree for simple XML documents, lxml for advanced features and performance, and xml.dom.minidom for detailed manipulation and formatting.
- Validate XML Documents
Validating XML documents against a schema ensures data integrity and consistency. Use libraries like lxml or external tools to validate XML files against XML Schema (XSD) or Document Type Definition (DTD).
- Handle XML Namespaces
XML namespaces prevent element name conflicts in XML documents. When working with XML documents that use namespaces, ensure that your code correctly handles them by using qualified names and the appropriate methods provided by the library.
- Optimize Performance
For large XML documents, optimize performance by using efficient parsing techniques. Libraries like lxml offer better performance for large files. Additionally, consider streaming parsers like xml.sax
for memory-efficient processing of large XML files.
- Maintain Readability
Maintain code readability by using clear variable names, comments, and proper indentation. When creating XML documents, use pretty-printing to make the output human-readable.
By following these best practices, developers can effectively process XML data in Python, ensuring robust and maintainable code.
相关问答FAQs:
如何使用Python创建XML文件?
使用Python创建XML文件可以通过标准库中的xml.etree.ElementTree
模块来实现。首先,需要导入该模块,然后构建XML的根元素和子元素。最后,使用ElementTree
的write
方法将XML树写入文件中。例如,可以这样构建一个简单的XML结构并保存到文件:
import xml.etree.ElementTree as ET
root = ET.Element("根元素")
子元素 = ET.SubElement(root, "子元素")
子元素.text = "这里是子元素的内容"
树 = ET.ElementTree(root)
树.write("输出.xml", encoding='utf-8', xml_declaration=True)
Python中有哪些库可以用来处理XML数据?
Python提供了多种库来处理XML数据。除了xml.etree.ElementTree
,还有minidom
、lxml
和xmltodict
等。minidom
适合用于创建和解析小型XML文档,而lxml
提供了更强大的功能和更高的性能,适合处理复杂的XML数据。xmltodict
则允许将XML数据转换为Python字典,方便进行数据处理和访问。
在Python中读取XML文件的步骤是什么?
在Python中读取XML文件通常需要使用xml.etree.ElementTree
模块。可以通过parse
方法加载XML文件,并使用getroot
方法获取根元素。接下来,可以遍历子元素,提取所需的信息。以下是一个简单的示例:
import xml.etree.ElementTree as ET
树 = ET.parse('输入.xml')
根 = 树.getroot()
for 子元素 in 根:
print(子元素.tag, 子元素.text)
这种方法使得提取和操作XML数据变得非常简单和高效。
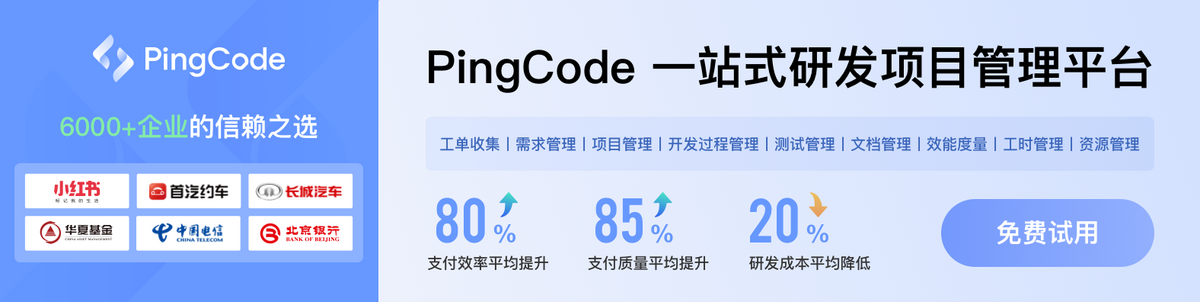