在Python中验证代理IP的有效性可以通过多种方法实现。通常的做法包括:发送HTTP请求、检查响应状态码、使用第三方库如requests
和urllib
进行代理设置、检测返回的IP地址。其中,发送HTTP请求并检查响应状态码是最常用的方法之一,因为它可以快速确认代理的可用性。接下来,我们将详细探讨如何在Python中实现这些方法。
一、发送HTTP请求验证代理IP
发送HTTP请求是验证代理IP有效性的一种直接方法。在请求过程中,我们需要设置代理IP,并通过捕获异常来确定代理是否可用。
- 使用
requests
库验证代理IP
requests
库是Python中一个非常流行的HTTP库,它支持代理设置。通过requests
库,我们可以很方便地发送带有代理的HTTP请求。
import requests
def is_proxy_working(proxy):
try:
# 设置代理
proxies = {
"http": proxy,
"https": proxy
}
# 发送HTTP请求
response = requests.get("http://httpbin.org/ip", proxies=proxies, timeout=5)
# 检查响应状态码
if response.status_code == 200:
print(f"Proxy {proxy} is working. Response IP: {response.json()}")
return True
else:
print(f"Proxy {proxy} failed. Status code: {response.status_code}")
return False
except requests.exceptions.RequestException as e:
print(f"Proxy {proxy} failed. Error: {e}")
return False
示例
proxy = "http://123.456.789.012:8080"
is_proxy_working(proxy)
- 使用
urllib
库验证代理IP
urllib
是Python的标准库,同样可以用于代理设置和HTTP请求。使用urllib
时,我们需要手动设置代理并处理异常。
import urllib.request
def is_proxy_working(proxy):
try:
# 设置代理
proxy_handler = urllib.request.ProxyHandler({'http': proxy, 'https': proxy})
opener = urllib.request.build_opener(proxy_handler)
urllib.request.install_opener(opener)
# 发送HTTP请求
response = urllib.request.urlopen("http://httpbin.org/ip", timeout=5)
# 检查响应状态码
if response.status == 200:
print(f"Proxy {proxy} is working. Response IP: {response.read().decode('utf-8')}")
return True
else:
print(f"Proxy {proxy} failed. Status code: {response.status}")
return False
except urllib.error.URLError as e:
print(f"Proxy {proxy} failed. Error: {e}")
return False
示例
proxy = "http://123.456.789.012:8080"
is_proxy_working(proxy)
二、检测返回的IP地址
为了确保代理IP的匿名性或验证其真实性,我们可以对返回的IP地址进行检测。通常情况下,我们通过访问一个返回请求IP的服务来获取当前的IP地址。
- 使用
httpbin.org
获取请求IP
httpbin.org
是一个简单的HTTP请求和响应服务,可以返回请求的IP地址。我们可以通过访问http://httpbin.org/ip
来获取IP信息。
import requests
def get_ip_via_proxy(proxy):
try:
proxies = {
"http": proxy,
"https": proxy
}
response = requests.get("http://httpbin.org/ip", proxies=proxies, timeout=5)
if response.status_code == 200:
return response.json().get('origin')
else:
return None
except requests.exceptions.RequestException:
return None
示例
proxy = "http://123.456.789.012:8080"
proxy_ip = get_ip_via_proxy(proxy)
if proxy_ip:
print(f"Accessed via proxy IP: {proxy_ip}")
else:
print("Failed to access via proxy.")
- 使用其他在线服务获取请求IP
除了httpbin.org
,还有许多其他的在线服务可以返回请求的IP地址,比如ipinfo.io
、icanhazip.com
等。使用这些服务的方式与httpbin.org
类似。
import requests
def get_ip_via_proxy(proxy, service_url):
try:
proxies = {
"http": proxy,
"https": proxy
}
response = requests.get(service_url, proxies=proxies, timeout=5)
if response.status_code == 200:
return response.text.strip()
else:
return None
except requests.exceptions.RequestException:
return None
示例
proxy = "http://123.456.789.012:8080"
service_url = "http://icanhazip.com"
proxy_ip = get_ip_via_proxy(proxy, service_url)
if proxy_ip:
print(f"Accessed via proxy IP: {proxy_ip}")
else:
print("Failed to access via proxy.")
三、检查响应时间
代理的响应时间是评估其性能的重要指标。我们可以通过记录请求的开始和结束时间来计算代理的响应时间。
- 使用
time
模块记录响应时间
通过time
模块,我们可以轻松记录发送请求前后的时间差,以此计算响应时间。
import requests
import time
def check_proxy_response_time(proxy):
try:
proxies = {
"http": proxy,
"https": proxy
}
start_time = time.time()
response = requests.get("http://httpbin.org/ip", proxies=proxies, timeout=5)
end_time = time.time()
if response.status_code == 200:
response_time = end_time - start_time
print(f"Proxy {proxy} is working. Response time: {response_time:.2f} seconds.")
return response_time
else:
print(f"Proxy {proxy} failed. Status code: {response.status_code}")
return None
except requests.exceptions.RequestException as e:
print(f"Proxy {proxy} failed. Error: {e}")
return None
示例
proxy = "http://123.456.789.012:8080"
response_time = check_proxy_response_time(proxy)
if response_time is not None:
print(f"Proxy response time: {response_time:.2f} seconds.")
else:
print("Failed to measure proxy response time.")
- 设定响应时间阈值
为了筛选出性能较好的代理,我们可以设定一个响应时间阈值。只有在响应时间低于该阈值时,代理才被认为是可用的。
def is_proxy_fast_enough(proxy, threshold=2.0):
response_time = check_proxy_response_time(proxy)
if response_time is not None and response_time < threshold:
print(f"Proxy {proxy} is fast enough. Response time: {response_time:.2f} seconds.")
return True
else:
print(f"Proxy {proxy} is too slow or not working.")
return False
示例
proxy = "http://123.456.789.012:8080"
is_fast = is_proxy_fast_enough(proxy, threshold=1.5)
if is_fast:
print("Proxy meets the performance requirements.")
else:
print("Proxy does not meet the performance requirements.")
四、批量验证代理IP
当我们需要验证多个代理IP时,可以通过批量处理的方式提高效率。我们可以使用多线程或异步编程来加速验证过程。
- 使用多线程进行批量验证
通过concurrent.futures
模块中的ThreadPoolExecutor
,我们可以轻松实现多线程批量验证。
import requests
import concurrent.futures
def is_proxy_working(proxy):
try:
proxies = {
"http": proxy,
"https": proxy
}
response = requests.get("http://httpbin.org/ip", proxies=proxies, timeout=5)
return response.status_code == 200
except requests.exceptions.RequestException:
return False
def batch_verify_proxies(proxies):
with concurrent.futures.ThreadPoolExecutor() as executor:
results = executor.map(is_proxy_working, proxies)
return list(results)
示例
proxy_list = ["http://123.456.789.012:8080", "http://234.567.890.123:8080"]
working_proxies = batch_verify_proxies(proxy_list)
print(f"Working proxies: {working_proxies}")
- 使用异步编程进行批量验证
异步编程是另一种高效处理大量请求的方法。通过asyncio
和aiohttp
库,我们可以实现异步的代理验证。
import aiohttp
import asyncio
async def is_proxy_working(session, proxy):
try:
async with session.get("http://httpbin.org/ip", proxy=proxy, timeout=5) as response:
return response.status == 200
except:
return False
async def batch_verify_proxies(proxies):
async with aiohttp.ClientSession() as session:
tasks = [is_proxy_working(session, proxy) for proxy in proxies]
results = await asyncio.gather(*tasks)
return results
示例
proxy_list = ["http://123.456.789.012:8080", "http://234.567.890.123:8080"]
loop = asyncio.get_event_loop()
working_proxies = loop.run_until_complete(batch_verify_proxies(proxy_list))
print(f"Working proxies: {working_proxies}")
五、总结
验证代理IP的有效性是使用代理服务中的一个重要步骤。通过发送HTTP请求、检测返回的IP地址、检查响应时间等方法,我们可以有效地验证代理的可用性和性能。此外,批量验证的实现使得我们能够更高效地处理多个代理IP。无论是通过多线程还是异步编程,我们都可以显著提高代理验证的效率。
在实际应用中,选择合适的方法和工具至关重要。requests
库以其简单易用的接口成为大多数开发者的首选,而对于更高性能的需求,asyncio
和aiohttp
提供了强大的异步处理能力。通过合理的工具组合,我们可以确保代理IP的有效性和可靠性,从而在各类应用中取得更好的效果。
相关问答FAQs:
如何判断一个代理IP是否有效?
要判断一个代理IP是否有效,可以通过尝试使用该IP进行网络请求。可以使用Python的requests库来发送请求,观察返回的状态码和响应时间。如果请求成功且状态码为200,说明代理IP有效。此外,记录请求的延迟时间也可以帮助判断代理的性能。
使用Python验证代理IP时应该注意哪些事项?
在验证代理IP时,需要考虑几个因素。首先,确保代理IP的类型(如HTTP、HTTPS或SOCKS)与您使用的请求方式相匹配。其次,注意代理的匿名级别,不同级别的代理对请求的隐私保护程度不同。最后,选择一个合适的验证方法,例如使用多个目标网站进行测试,以确保代理的稳定性和可靠性。
有哪些库可以用来验证代理IP?
Python中有多个库可以用来验证代理IP,最常用的包括requests、httpx和aiohttp。requests库适合于简单的同步请求,而httpx和aiohttp则支持异步请求,适合需要高并发验证的场景。此外,还有一些专门的库如PyProxy和ProxyBroker,可以帮助用户更方便地管理和验证代理IP。
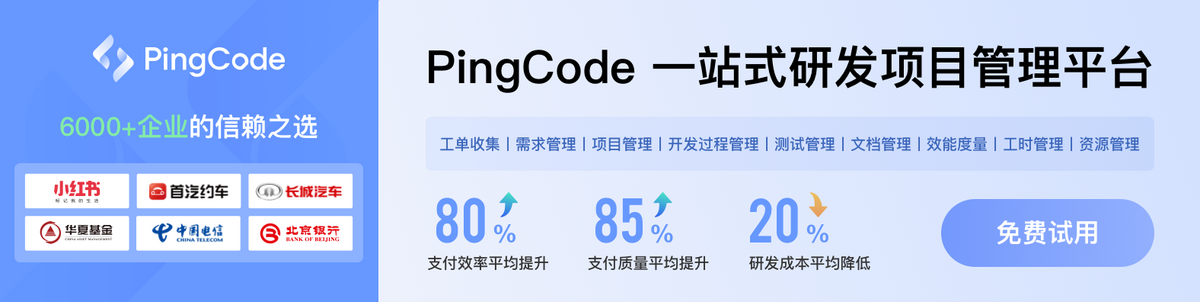