在Python中消除字符串中的空格,可以使用以下几种方法:strip()方法、replace()方法、split()和join()方法组合。其中,strip()
方法用于去除字符串两端的空格,replace()
方法可以用来替换所有空格,而split()
和join()
方法组合使用可以去除字符串中的所有空格并保留单词的间隔。接下来,我将详细介绍这些方法。
一、使用STRIP()方法消除空格
strip()
方法用于去除字符串两端的空格。这在需要清除用户输入时特别有用。strip()
不会影响字符串中间的空格。
-
基本用法
使用
strip()
方法可以很简单地去除字符串开头和结尾的空格。例如:my_string = " Hello, World! "
cleaned_string = my_string.strip()
print(cleaned_string) # 输出: "Hello, World!"
-
去除特定字符
除了空格,
strip()
还可以去除指定的字符集。例如:my_string = "---Hello, World!---"
cleaned_string = my_string.strip('-')
print(cleaned_string) # 输出: "Hello, World!"
二、使用REPLACE()方法消除空格
replace()
方法可以用来替换字符串中的子字符串。通过将空格替换为空字符串,可以去除所有空格。
-
消除所有空格
使用
replace()
方法替换空格为无字符字符串(即删除空格):my_string = "Hello, World!"
no_spaces = my_string.replace(" ", "")
print(no_spaces) # 输出: "Hello,World!"
-
替换特定空白
也可以使用
replace()
方法替换特定的字符或子字符串。例如:my_string = "Hello, World!"
single_space = my_string.replace(" ", " ")
print(single_space) # 输出: "Hello, World!"
三、使用SPLIT()和JOIN()方法组合
通过结合split()
和join()
,可以有效地去除字符串中的多余空格,保留单词间的单一空格。
-
消除多余空格
这对需要清理文本数据,确保单词之间只有一个空格特别有用:
my_string = "Hello, World! How are you?"
split_string = my_string.split()
cleaned_string = " ".join(split_string)
print(cleaned_string) # 输出: "Hello, World! How are you?"
-
处理换行和制表符
split()
方法默认会去除所有的空白字符,包括换行符和制表符,这使它成为处理多行文本的好工具:my_string = "Hello,\nWorld!\tHow are you?"
split_string = my_string.split()
cleaned_string = " ".join(split_string)
print(cleaned_string) # 输出: "Hello, World! How are you?"
四、应用场景与注意事项
-
用户输入处理
在开发用户交互的应用程序时,清除用户输入中的多余空格是很常见的需求。无论是登录系统,还是表单提交,用户的输入往往会带有不必要的空格。通过使用
strip()
或replace()
,可以确保数据的一致性和准确性。 -
数据预处理
数据分析和机器学习任务中,预处理数据时常需要消除数据中的空格。空格可能会导致数据解析错误或者不准确的分析结果。
split()
和join()
的组合使用可以帮助清理文本数据。 -
性能考虑
在处理大规模文本数据时,选择合适的方法可以提高程序的效率。例如,
replace()
方法在需要去除所有空格时非常有效,而split()
和join()
方法则适合在需要保持单词间单一空格时使用。
通过熟练应用这些方法,开发人员可以有效地清理和处理字符串中的空格,从而提高程序的健壮性和数据的准确性。
相关问答FAQs:
如何在Python中删除字符串中的空格?
在Python中,删除字符串中的空格可以通过多种方法实现。最简单的方式是使用str.replace()
方法,您可以将空格替换为空字符串。示例代码如下:
string_with_spaces = " Hello, World! "
cleaned_string = string_with_spaces.replace(" ", "")
这样,cleaned_string
将为"Hello,World!"
。
使用Python的strip()方法有什么优势?strip()
方法可以删除字符串开头和结尾的空格。这对于处理用户输入或者清理数据时非常有用。使用示例:
user_input = " Hello! "
cleaned_input = user_input.strip()
结果是"Hello!"
,这个方法不会影响字符串中间的空格。
如何使用正则表达式消除字符串中的所有空格?
使用Python的re
模块,您可以通过正则表达式来删除字符串中的所有空格。示例代码如下:
import re
string_with_spaces = " Hello, World! "
cleaned_string = re.sub(r'\s+', '', string_with_spaces)
这样,您可以有效地去除字符串中的所有空格,包括连续的空格,得到"Hello,World!"
。
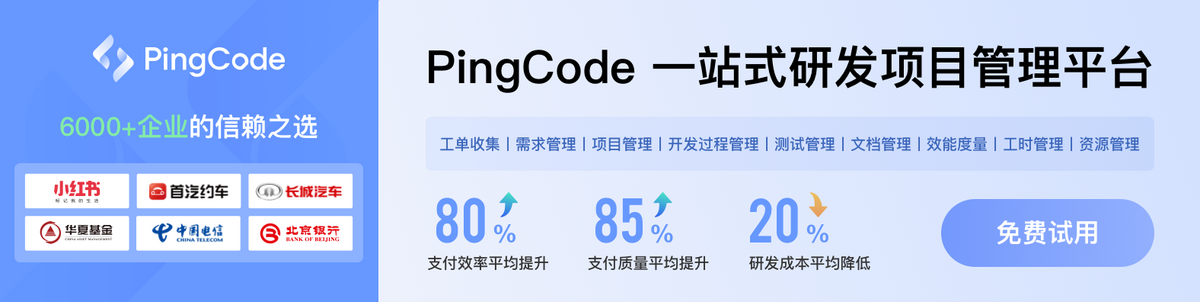