一、引言
用Python编写婚礼请柬可以通过使用现有的库如PIL
(Pillow)、ReportLab
、Fpdf
等来实现,轻松生成个性化、精美的请柬、通过编程生成请柬具有高效率、易于修改、自动化处理等优势。在本篇文章中,我们将详细介绍如何使用Python编写一个婚礼请柬,包括从基础的文本处理到添加图片、设计版式等各个方面。
二、选择合适的库
在开始编写婚礼请柬之前,首先需要选择合适的库来处理图像和文本。Python提供了多种库可以实现这一功能:
1、Pillow (PIL)
Pillow是Python图像库的一个分支,功能强大且易于使用。它可以处理图像的打开、修改和保存等操作。
from PIL import Image, ImageDraw, ImageFont
2、ReportLab
ReportLab是一个用于生成PDF文档的强大库,它可以帮助我们轻松创建复杂的文档。
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
3、FPDF
FPDF是一个轻量级的PDF生成库,适用于简单的PDF文档生成。
from fpdf import FPDF
三、设计请柬的基本结构
在开始编写代码之前,首先要设计请柬的基本结构。请柬通常包括以下几个部分:
1、标题
请柬的标题通常是新人的名字和婚礼日期。
2、正文
正文部分通常包括婚礼的具体时间、地点以及其他重要信息。
3、装饰图案
装饰图案可以是一些婚礼相关的图片,如花朵、戒指等。
四、使用Pillow生成请柬
1、安装Pillow库
首先,确保安装了Pillow库,可以使用以下命令进行安装:
pip install pillow
2、编写代码生成请柬
下面是一个简单的示例代码,展示了如何使用Pillow库生成一个婚礼请柬:
from PIL import Image, ImageDraw, ImageFont
创建一个空白图像
width, height = 800, 600
image = Image.new('RGB', (width, height), 'white')
创建一个绘图对象
draw = ImageDraw.Draw(image)
加载字体
font_path = "/usr/share/fonts/truetype/dejavu/DejaVuSans-Bold.ttf"
title_font = ImageFont.truetype(font_path, 40)
body_font = ImageFont.truetype(font_path, 20)
绘制标题
title_text = "John & Jane's Wedding"
title_width, title_height = draw.textsize(title_text, font=title_font)
title_position = ((width - title_width) / 2, 50)
draw.text(title_position, title_text, fill="black", font=title_font)
绘制正文
body_text = (
"We are delighted to invite you to our wedding.\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
)
body_width, body_height = draw.textsize(body_text, font=body_font)
body_position = ((width - body_width) / 2, 150)
draw.text(body_position, body_text, fill="black", font=body_font)
保存图像
image.save("wedding_invitation.png")
五、使用ReportLab生成PDF请柬
1、安装ReportLab库
首先,确保安装了ReportLab库,可以使用以下命令进行安装:
pip install reportlab
2、编写代码生成PDF请柬
下面是一个简单的示例代码,展示了如何使用ReportLab库生成一个婚礼请柬:
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
创建一个PDF文件
pdf_filename = "wedding_invitation.pdf"
c = canvas.Canvas(pdf_filename, pagesize=letter)
设置标题
c.setFont("Helvetica-Bold", 40)
c.drawCentredString(300, 750, "John & Jane's Wedding")
设置正文
c.setFont("Helvetica", 20)
text = (
"We are delighted to invite you to our wedding.\n\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
)
c.drawString(100, 700, text)
保存PDF文件
c.save()
六、使用FPDF生成PDF请柬
1、安装FPDF库
首先,确保安装了FPDF库,可以使用以下命令进行安装:
pip install fpdf
2、编写代码生成PDF请柬
下面是一个简单的示例代码,展示了如何使用FPDF库生成一个婚礼请柬:
from fpdf import FPDF
创建一个PDF对象
pdf = FPDF()
添加一页
pdf.add_page()
设置标题
pdf.set_font("Arial", 'B', 16)
pdf.cell(200, 10, "John & Jane's Wedding", ln=True, align='C')
设置正文
pdf.set_font("Arial", size=12)
pdf.multi_cell(0, 10, (
"We are delighted to invite you to our wedding.\n\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
))
保存PDF文件
pdf.output("wedding_invitation.pdf")
七、添加更多设计元素
1、添加图片
在请柬中添加图片可以使其更具个性化和吸引力。在Pillow中,可以使用Image.paste
方法添加图片。在ReportLab中,可以使用drawImage
方法。在FPDF中,可以使用image
方法。
示例(Pillow):
# 打开图片
flower_image = Image.open("flower.png")
调整图片大小
flower_image = flower_image.resize((100, 100))
粘贴图片到请柬
image.paste(flower_image, (350, 300))
示例(ReportLab):
# 添加图片到PDF
c.drawImage("flower.png", 250, 500, width=100, height=100)
示例(FPDF):
# 添加图片到PDF
pdf.image("flower.png", x=100, y=100, w=100, h=100)
2、调整字体和颜色
不同的字体和颜色可以使请柬更加美观。在Pillow中,可以使用ImageFont.truetype
方法加载不同的字体,并使用fill
参数设置颜色。在ReportLab中,可以使用setFont
和setFillColor
方法。在FPDF中,可以使用set_font
和set_text_color
方法。
示例(Pillow):
# 加载不同的字体
title_font = ImageFont.truetype("Arial-Bold.ttf", 50)
body_font = ImageFont.truetype("Arial.ttf", 25)
设置颜色
draw.text(title_position, title_text, fill="blue", font=title_font)
draw.text(body_position, body_text, fill="green", font=body_font)
示例(ReportLab):
# 设置不同的字体和颜色
c.setFont("Times-Bold", 50)
c.setFillColorRGB(0, 0, 1) # Blue color
c.drawCentredString(300, 750, "John & Jane's Wedding")
c.setFont("Times-Roman", 25)
c.setFillColorRGB(0, 1, 0) # Green color
c.drawString(100, 700, text)
示例(FPDF):
# 设置不同的字体和颜色
pdf.set_font("Arial", 'B', 20)
pdf.set_text_color(0, 0, 255) # Blue color
pdf.cell(200, 10, "John & Jane's Wedding", ln=True, align='C')
pdf.set_font("Arial", size=15)
pdf.set_text_color(0, 255, 0) # Green color
pdf.multi_cell(0, 10, text)
八、结论
通过使用Python和上述提到的库,我们可以轻松编写一个婚礼请柬。使用编程生成请柬不仅可以提高效率,还可以实现高度的个性化和自动化处理。无论是选择Pillow、ReportLab还是FPDF,每个库都有其独特的优势,选择合适的工具可以使开发过程更加顺利。希望这篇文章能为你提供有价值的参考,并帮助你成功创建美观且个性化的婚礼请柬。
九、附录:完整代码示例
1、Pillow完整代码
from PIL import Image, ImageDraw, ImageFont
创建一个空白图像
width, height = 800, 600
image = Image.new('RGB', (width, height), 'white')
创建一个绘图对象
draw = ImageDraw.Draw(image)
加载字体
font_path = "path/to/your/font.ttf"
title_font = ImageFont.truetype(font_path, 40)
body_font = ImageFont.truetype(font_path, 20)
绘制标题
title_text = "John & Jane's Wedding"
title_width, title_height = draw.textsize(title_text, font=title_font)
title_position = ((width - title_width) / 2, 50)
draw.text(title_position, title_text, fill="black", font=title_font)
绘制正文
body_text = (
"We are delighted to invite you to our wedding.\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
)
body_width, body_height = draw.textsize(body_text, font=body_font)
body_position = ((width - body_width) / 2, 150)
draw.text(body_position, body_text, fill="black", font=body_font)
打开并粘贴装饰图片
flower_image = Image.open("path/to/flower.png")
flower_image = flower_image.resize((100, 100))
image.paste(flower_image, (350, 300))
保存图像
image.save("wedding_invitation.png")
2、ReportLab完整代码
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
创建一个PDF文件
pdf_filename = "wedding_invitation.pdf"
c = canvas.Canvas(pdf_filename, pagesize=letter)
设置标题
c.setFont("Helvetica-Bold", 40)
c.drawCentredString(300, 750, "John & Jane's Wedding")
设置正文
c.setFont("Helvetica", 20)
text = (
"We are delighted to invite you to our wedding.\n\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
)
c.drawString(100, 700, text)
添加装饰图片
c.drawImage("path/to/flower.png", 250, 500, width=100, height=100)
保存PDF文件
c.save()
3、FPDF完整代码
from fpdf import FPDF
创建一个PDF对象
pdf = FPDF()
添加一页
pdf.add_page()
设置标题
pdf.set_font("Arial", 'B', 16)
pdf.cell(200, 10, "John & Jane's Wedding", ln=True, align='C')
设置正文
pdf.set_font("Arial", size=12)
pdf.multi_cell(0, 10, (
"We are delighted to invite you to our wedding.\n\n"
"Date: 25th December 2023\n"
"Time: 4:00 PM\n"
"Venue: St. Mary's Church\n"
))
添加装饰图片
pdf.image("path/to/flower.png", x=100, y=100, w=100, h=100)
保存PDF文件
pdf.output("wedding_invitation.pdf")
通过这些示例代码,你可以轻松地创建个性化的婚礼请柬,并根据需要进行进一步定制。希望这些内容能帮助你顺利完成婚礼请柬的制作。
相关问答FAQs:
如何用Python生成婚礼请柬的设计模板?
要用Python生成婚礼请柬的设计模板,您可以使用PIL(Python Imaging Library)或其分支Pillow库。首先,安装Pillow库:pip install Pillow
。然后,您可以创建一个新的图像,添加背景、文字和装饰元素,最终保存成图片格式。示例代码如下:
from PIL import Image, ImageDraw, ImageFont
# 创建一个白色背景的图像
image = Image.new('RGB', (800, 600), 'white')
draw = ImageDraw.Draw(image)
# 添加文本
font = ImageFont.truetype("arial.ttf", 40)
draw.text((100, 100), "邀请您参加我们的婚礼", fill="black", font=font)
# 保存图像
image.save('wedding_invitation.png')
通过调整字体、颜色和布局,您可以设计出独特的婚礼请柬。
可以使用Python生成电子请柬吗?
是的,Python可以生成电子请柬,您可以使用Flask或Django等Web框架创建一个简单的应用程序,让用户输入婚礼信息。将这些信息渲染为HTML模板并生成PDF或图像文件供分享。使用weasyprint
库可以将HTML转换为PDF,简单且高效。
有哪些Python库可以帮助设计婚礼请柬的图形元素?
除了PIL/Pillow,您还可以使用Matplotlib、OpenCV或ReportLab等库来设计婚礼请柬的图形元素。Matplotlib适合生成图表和简单图形,而OpenCV更适合图像处理,ReportLab则专注于PDF文档的生成。根据您的需求选择合适的库,可以帮助您创造出更具吸引力的请柬设计。
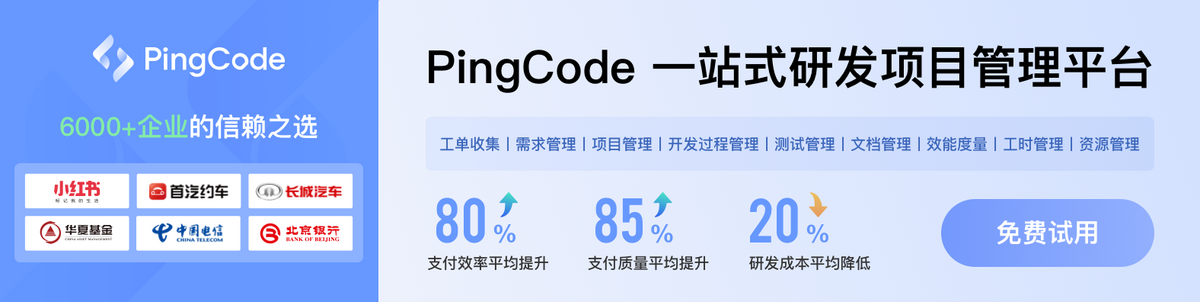