Python循环爬取数据的方法包括:使用for循环、while循环、结合时间间隔进行爬取。可以利用Scrapy框架进行循环爬取。
一、使用for循环进行数据爬取
for循环是Python中最常见的循环结构之一,可以非常方便地用于循环爬取数据。以下是一个简单的示例,演示如何使用for循环爬取多个网页的数据。
import requests
from bs4 import BeautifulSoup
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 处理页面内容
print(soup.title.text)
else:
print(f"Failed to retrieve {url}")
在这个示例中,我们定义了一个包含多个URL的列表,然后使用for循环遍历这些URL,依次发送HTTP请求并处理响应。BeautifulSoup是一个用于解析HTML和XML的库,可以方便地提取页面中的数据。
二、使用while循环进行数据爬取
while循环也是一种常见的循环结构,适用于需要根据特定条件持续爬取数据的场景。以下是一个示例,演示如何使用while循环爬取分页数据。
import requests
from bs4 import BeautifulSoup
爬取分页数据
base_url = 'https://example.com/page'
page = 1
while True:
url = f"{base_url}{page}"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 处理页面内容
print(soup.title.text)
page += 1
# 判断是否到达最后一页(这里假设存在一个条件判断)
if not soup.find('a', text='Next'):
break
else:
print(f"Failed to retrieve {url}")
break
在这个示例中,我们使用while循环不断地发送HTTP请求,直到到达最后一页。通过检查页面中是否存在“下一页”链接,我们可以确定何时停止爬取。
三、结合时间间隔进行爬取
为了避免被网站屏蔽或者减少服务器压力,我们可以在循环中加入时间间隔。以下是一个示例,演示如何结合时间间隔进行数据爬取。
import requests
from bs4 import BeautifulSoup
import time
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 处理页面内容
print(soup.title.text)
else:
print(f"Failed to retrieve {url}")
# 等待2秒钟
time.sleep(2)
在这个示例中,我们在每次爬取后使用time.sleep(2)暂停2秒钟,以避免对服务器造成过大的压力。
四、利用Scrapy框架进行循环爬取
Scrapy是一个功能强大的Python爬虫框架,适用于复杂的爬取任务。以下是一个示例,演示如何使用Scrapy进行循环爬取。
首先,安装Scrapy:
pip install scrapy
然后,创建一个新的Scrapy项目:
scrapy startproject myproject
接下来,创建一个新的爬虫:
cd myproject
scrapy genspider myspider example.com
编辑生成的myspider.py
文件,添加爬取逻辑:
import scrapy
class MySpider(scrapy.Spider):
name = "myspider"
start_urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
def parse(self, response):
# 处理页面内容
self.log(response.css('title::text').get())
# 爬取下一页(如果有的话)
next_page = response.css('a.next::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
最后,运行爬虫:
scrapy crawl myspider
在这个示例中,我们定义了一个Scrapy爬虫,通过设置start_urls来指定起始URL。parse方法用于处理响应,并提取页面数据。通过response.follow方法,我们可以递归地爬取下一页的数据。
五、处理动态网页
有些网页使用JavaScript动态加载内容,这时候我们需要使用像Selenium这样的工具来模拟浏览器行为。以下是一个示例,演示如何使用Selenium爬取动态网页的数据。
首先,安装Selenium和浏览器驱动(例如ChromeDriver):
pip install selenium
接着,编写爬取逻辑:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
设置ChromeDriver路径
driver_path = '/path/to/chromedriver'
启动浏览器
driver = webdriver.Chrome(executable_path=driver_path)
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
driver.get(url)
time.sleep(2) # 等待页面加载
# 处理页面内容
title = driver.find_element(By.TAG_NAME, 'title').text
print(title)
关闭浏览器
driver.quit()
在这个示例中,我们使用Selenium启动一个Chrome浏览器实例,并访问多个网页。通过find_element方法,我们可以提取页面中的数据。
六、处理反爬机制
许多网站都有反爬机制,如验证码、IP封禁、请求频率限制等。以下是一些常见的应对策略:
- 使用代理:通过使用代理IP,可以避免同一个IP频繁访问导致被封禁。
- 模拟浏览器行为:通过设置请求头、使用Selenium等工具,可以模拟真实用户的浏览器行为。
- 处理验证码:可以使用OCR技术识别验证码,或者通过人工输入解决。
- 设置合理的爬取间隔:通过设置爬取间隔,减少对服务器的压力,避免触发反爬机制。
以下是一个使用代理的示例:
import requests
from bs4 import BeautifulSoup
设置代理
proxies = {
'http': 'http://user:pass@proxyserver:port',
'https': 'https://user:pass@proxyserver:port',
}
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 处理页面内容
print(soup.title.text)
else:
print(f"Failed to retrieve {url}")
在这个示例中,我们通过设置proxies参数使用代理IP进行爬取。
七、保存爬取的数据
爬取的数据通常需要保存到文件、数据库或其他存储介质中。以下是一些常见的数据保存方法:
- 保存到文件:可以使用Python的内置文件操作函数保存数据到文本文件、CSV文件等。
- 保存到数据库:可以使用SQLite、MySQL、MongoDB等数据库保存数据。
- 使用Scrapy的Item Pipeline:在Scrapy中,可以通过Item Pipeline将数据保存到文件、数据库等。
以下是一个保存数据到CSV文件的示例:
import requests
from bs4 import BeautifulSoup
import csv
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
打开CSV文件
with open('data.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title'])
for url in urls:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 提取页面数据
title = soup.title.text
writer.writerow([title])
else:
print(f"Failed to retrieve {url}")
在这个示例中,我们使用csv库将爬取的数据保存到CSV文件中。
八、数据清洗和处理
爬取的数据通常需要进行清洗和处理,以便后续分析和使用。以下是一些常见的数据清洗和处理方法:
- 去除HTML标签:可以使用BeautifulSoup、正则表达式等去除HTML标签。
- 处理缺失值:可以使用填充、删除等方法处理缺失值。
- 数据转换:可以将数据转换为适当的格式,如日期、数值等。
以下是一个去除HTML标签的示例:
import requests
from bs4 import BeautifulSoup
import re
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# 提取并清洗页面数据
raw_text = soup.get_text()
clean_text = re.sub(r'\s+', ' ', raw_text)
print(clean_text)
else:
print(f"Failed to retrieve {url}")
在这个示例中,我们使用re.sub函数去除多余的空白字符,从而清洗页面数据。
九、并发爬取
为了提高爬取效率,我们可以使用多线程、多进程或异步IO进行并发爬取。以下是一些常见的并发爬取方法:
- 多线程:可以使用
threading
库进行多线程爬取。 - 多进程:可以使用
multiprocessing
库进行多进程爬取。 - 异步IO:可以使用
asyncio
库进行异步IO爬取。
以下是一个使用多线程进行并发爬取的示例:
import requests
from bs4 import BeautifulSoup
import threading
爬取多个网页的数据
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
def fetch_data(url):
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.title.text)
else:
print(f"Failed to retrieve {url}")
threads = []
for url in urls:
thread = threading.Thread(target=fetch_data, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
在这个示例中,我们使用threading.Thread
创建多个线程,并发地爬取多个网页的数据。
十、总结
通过上述方法,您可以使用Python循环爬取数据,并处理各种不同的需求和挑战。无论是简单的for循环、while循环,还是使用Scrapy框架、Selenium工具进行动态网页爬取,都可以满足不同场景下的数据爬取需求。
在实际应用中,您可能会遇到各种各样的问题,例如反爬机制、数据清洗、并发爬取等。通过掌握上述方法和技巧,您将能够更好地应对这些挑战,并高效地完成数据爬取任务。
相关问答FAQs:
如何使用Python进行循环爬取数据的基本步骤是什么?
在使用Python进行循环爬取数据时,首先需要选择合适的库,如requests
和BeautifulSoup
。接着,设置一个循环结构(如for
或while
)来多次发送请求。在每次请求中,解析返回的HTML内容,提取所需数据,并将其存储在列表或字典中。确保在请求之间设置适当的延时,以避免对目标网站造成过大压力。
在爬取数据时,如何处理网站的反爬虫机制?
为了应对网站的反爬虫机制,可以采取多种策略,例如随机更换请求头(User-Agent),使用代理IP来隐藏真实IP地址,以及增加请求间隔时间。此外,可以考虑使用模拟浏览器的工具,如Selenium
,以更真实的方式与网站交互,从而减少被封禁的风险。
如何确保循环爬取的数据的准确性和完整性?
确保数据准确性和完整性的方法包括:在爬取数据前,提前检查目标网页的结构,以便正确定位所需信息。同时,处理异常情况,使用try-except
结构捕获可能出现的错误,避免程序因单一错误而中断。定期对爬取的数据进行验证,确保其与网站上的实际内容相符。
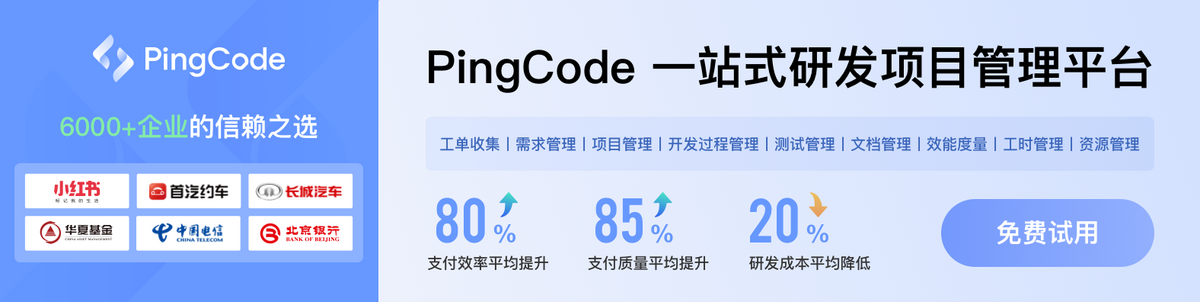