在Python中,str
是用于处理和操作字符串的内置数据类型。字符串是在单引号、双引号或三引号之间的字符序列。字符串是不可变的、支持各种方法和操作、可以通过索引和切片来访问字符。下面将详细介绍Python中字符串的工作原理及其相关操作。
一、字符串的定义与基本操作
Python中的字符串可以通过单引号、双引号或三引号来定义。例如:
single_quoted = 'Hello, World!'
double_quoted = "Hello, World!"
triple_quoted = '''Hello,
World!'''
字符串是不可变的,这意味着一旦创建了一个字符串,就无法修改它的内容。任何对字符串的修改操作都会创建一个新的字符串对象。例如:
original = "Hello"
modified = original + ", World!"
print(original) # 输出: Hello
print(modified) # 输出: Hello, World!
尽管我们对original
进行了拼接操作,但original
本身并没有改变。
二、字符串的方法与操作
Python内置了许多用于操作字符串的方法。以下是一些常用的方法:
-
字符串拼接与重复
- 使用
+
进行拼接
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # 输出: Hello World
- 使用
*
进行重复
repeated = "Hello" * 3
print(repeated) # 输出: HelloHelloHello
- 使用
-
字符串的拆分与连接
- 使用
split()
方法将字符串拆分为列表
sentence = "Hello World"
words = sentence.split()
print(words) # 输出: ['Hello', 'World']
- 使用
join()
方法将列表连接为字符串
words = ['Hello', 'World']
sentence = ' '.join(words)
print(sentence) # 输出: Hello World
- 使用
-
字符串的查找与替换
- 使用
find()
方法查找子字符串的位置
text = "Hello, World!"
position = text.find("World")
print(position) # 输出: 7
- 使用
replace()
方法替换子字符串
text = "Hello, World!"
new_text = text.replace("World", "Python")
print(new_text) # 输出: Hello, Python!
- 使用
-
字符串的大小写转换
- 使用
upper()
方法将字符串转换为大写
text = "Hello, World!"
uppercase_text = text.upper()
print(uppercase_text) # 输出: HELLO, WORLD!
- 使用
lower()
方法将字符串转换为小写
text = "Hello, World!"
lowercase_text = text.lower()
print(lowercase_text) # 输出: hello, world!
- 使用
三、字符串的索引与切片
字符串中的每个字符都有一个索引,索引从0开始。可以使用索引访问字符串中的单个字符。例如:
text = "Hello, World!"
first_character = text[0]
last_character = text[-1]
print(first_character) # 输出: H
print(last_character) # 输出: !
切片允许我们访问字符串的子字符串。切片的基本语法是[start:end:step]
。例如:
text = "Hello, World!"
substring = text[0:5]
print(substring) # 输出: Hello
在切片中,start
是起始索引,end
是结束索引(不包含),step
是步长。
四、字符串的格式化
Python提供了多种字符串格式化方法,使得在字符串中插入变量变得非常方便。其中包括旧式的%
格式化、新式的str.format()
方法以及最新的f-strings(格式化字符串字面量)。
-
百分号格式化
name = "Alice"
age = 25
formatted_string = "Name: %s, Age: %d" % (name, age)
print(formatted_string) # 输出: Name: Alice, Age: 25
-
str.format()
方法name = "Alice"
age = 25
formatted_string = "Name: {}, Age: {}".format(name, age)
print(formatted_string) # 输出: Name: Alice, Age: 25
-
f-strings(格式化字符串字面量)
name = "Alice"
age = 25
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string) # 输出: Name: Alice, Age: 25
五、字符串的编码与解码
字符串在内存中是以Unicode编码存储的,而在网络传输或文件存储时,通常需要将其编码为字节序列。Python中使用encode()
方法进行编码,使用decode()
方法进行解码。例如:
text = "Hello, World!"
encoded_text = text.encode('utf-8')
print(encoded_text) # 输出: b'Hello, World!'
decoded_text = encoded_text.decode('utf-8')
print(decoded_text) # 输出: Hello, World!
六、字符串的常见操作与技巧
-
去除字符串首尾的空白字符
- 使用
strip()
方法去除首尾空白
text = " Hello, World! "
stripped_text = text.strip()
print(stripped_text) # 输出: Hello, World!
- 使用
lstrip()
去除左侧空白,rstrip()
去除右侧空白
text = " Hello, World! "
left_stripped = text.lstrip()
right_stripped = text.rstrip()
print(left_stripped) # 输出: Hello, World!
print(right_stripped) # 输出: Hello, World!
- 使用
-
检查字符串是否以特定子字符串开头或结尾
- 使用
startswith()
和endswith()
方法
text = "Hello, World!"
starts_with_hello = text.startswith("Hello")
ends_with_world = text.endswith("World!")
print(starts_with_hello) # 输出: True
print(ends_with_world) # 输出: True
- 使用
-
字符串内的字符统计
- 使用
count()
方法统计子字符串出现的次数
text = "Hello, World! Hello, Python!"
count_hello = text.count("Hello")
print(count_hello) # 输出: 2
- 使用
-
检查字符串是否只包含字母或数字
- 使用
isalpha()
检查是否只包含字母,isdigit()
检查是否只包含数字,isalnum()
检查是否只包含字母和数字
text1 = "HelloWorld"
text2 = "12345"
text3 = "Hello123"
print(text1.isalpha()) # 输出: True
print(text2.isdigit()) # 输出: True
print(text3.isalnum()) # 输出: True
- 使用
七、字符串的高级操作
-
多行字符串与换行符
- 三引号可以定义多行字符串
multi_line_string = '''This is a
multi-line string.'''
print(multi_line_string)
- 使用
\n
表示换行符
single_line_with_newline = "This is a\nsingle line with a newline."
print(single_line_with_newline)
-
字符串的反转
- 可以使用切片操作反转字符串
text = "Hello, World!"
reversed_text = text[::-1]
print(reversed_text) # 输出: !dlroW ,olleH
-
字符串的内存效率
- 因为字符串是不可变的,所以频繁的字符串拼接会导致大量的内存开销。可以使用
str.join()
方法或其他高效的拼接方式来优化性能。
parts = ["Hello", "World", "Python"]
efficient_join = " ".join(parts)
print(efficient_join) # 输出: Hello World Python
- 因为字符串是不可变的,所以频繁的字符串拼接会导致大量的内存开销。可以使用
八、字符串与正则表达式
Python中的re
模块提供了强大的正则表达式功能,用于复杂的字符串匹配和操作。
-
使用正则表达式匹配模式
import re
text = "The rain in Spain"
match = re.search(r"\brain\b", text)
if match:
print("Match found!")
else:
print("No match found.")
-
使用正则表达式替换子字符串
import re
text = "The rain in Spain"
replaced_text = re.sub(r"rain", "snow", text)
print(replaced_text) # 输出: The snow in Spain
-
提取匹配的字符串
import re
text = "My phone number is 123-456-7890"
match = re.search(r"\d{3}-\d{3}-\d{4}", text)
if match:
print(f"Phone number found: {match.group()}")
九、字符串的国际化与本地化
在处理多语言和国际化应用时,字符串的国际化与本地化是非常重要的。Python提供了gettext
模块来支持这一点。
-
使用gettext模块
import gettext
gettext.bindtextdomain('myapp', 'locale')
gettext.textdomain('myapp')
_ = gettext.gettext
print(_("Hello, World!"))
-
使用多语言文件
- 创建.po文件和.mo文件来支持多语言
- 使用
xgettext
工具生成.po文件,并翻译后生成.mo文件
十、总结
通过对Python字符串的详细介绍,我们了解了字符串的定义、基本操作、方法和高级技巧。字符串是不可变的、支持各种方法和操作、可以通过索引和切片来访问字符。掌握这些知识能够帮助我们在实际编程中高效地处理和操作字符串。无论是简单的字符串拼接、查找与替换,还是复杂的正则表达式匹配和国际化应用,Python都提供了强大的工具和方法来满足我们的需求。
希望这篇文章能够帮助你更好地理解和使用Python中的字符串。
相关问答FAQs:
如何在Python中创建字符串?
在Python中,可以通过单引号、双引号或三重引号来创建字符串。例如,使用'Hello'
或"Hello"
创建简单字符串,而使用'''Hello'''
或"""Hello"""
可以创建多行字符串。Python会自动识别字符串的类型,并支持Unicode字符,使得处理不同语言的文本变得更加简单。
字符串在Python中有哪些常用的方法?
Python的字符串对象提供了许多内置方法。例如,str.upper()
可以将字符串转换为大写,str.lower()
用于小写转换,而str.replace(old, new)
可以替换字符串中的特定子串。其他有用的方法包括str.split(separator)
用于分割字符串,str.join(iterable)
用于将多个字符串连接成一个字符串。
如何在Python中格式化字符串?
Python提供了多种字符串格式化方法。使用f-string(格式化字符串字面量)可以通过在字符串前加f,并在花括号中放入变量名来实现,例如:name = "Alice"; greeting = f"Hello, {name}!"
。另外,str.format()
方法也可以用于格式化字符串,通过在字符串中使用花括号和指定参数来实现,例如:"Hello, {}".format(name)
。这些方法使得动态插入变量变得更加灵活和方便。
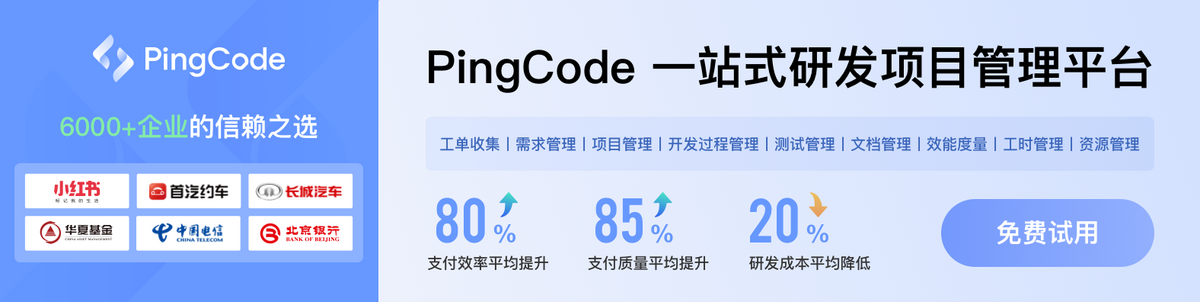