在 Python 中,打印当前行数可以通过使用内置模块 inspect
,该模块可以获取函数和方法的源代码行号。
我们可以使用 inspect.currentframe()
获取当前帧,然后使用 f_lineno
属性获取当前行号。例如:
import inspect
print("This is line number:", inspect.currentframe().f_lineno)
下面详细介绍如何在不同的情境下使用这个方法来获取和打印当前行数。
一、获取当前行数的方法
- 使用
inspect
模块
inspect
是 Python 标准库中的模块,可以提供对活动帧的访问。它主要用于检查活动帧、查找函数和方法的源代码行号以及调试目的。例如,下面的代码演示了如何使用 inspect
模块获取当前行号:
import inspect
def print_line_number():
print(f"Current line number: {inspect.currentframe().f_lineno}")
print_line_number()
在这个例子中,inspect.currentframe().f_lineno
获取了调用 print_line_number
函数时的当前行号。
- 使用
traceback
模块
traceback
模块提供了一种提取、格式化和打印堆栈跟踪的方式。通过 traceback.extract_stack()
方法可以获取当前堆栈,并且可以获取行号:
import traceback
def print_line_number():
stack = traceback.extract_stack()
_, _, _, line = stack[-2]
print(f"Current line number: {line}")
print_line_number()
在这个例子中,traceback.extract_stack()
返回了调用堆栈列表,stack[-2]
提供了调用 print_line_number
函数时的帧信息。
二、在不同情境下打印行号
- 在函数内部
在函数内部打印行号,可以使用上述任意一种方法。以下是一个示例:
import inspect
def my_function():
print(f"Line number inside function: {inspect.currentframe().f_lineno}")
# Other code
print(f"Line number inside function: {inspect.currentframe().f_lineno}")
my_function()
- 在类方法内部
在类方法内部打印行号,可以使用相同的方法:
import inspect
class MyClass:
def my_method(self):
print(f"Line number inside method: {inspect.currentframe().f_lineno}")
# Other code
print(f"Line number inside method: {inspect.currentframe().f_lineno}")
obj = MyClass()
obj.my_method()
- 在模块的全局范围
在模块的全局范围内打印行号,可以直接使用 inspect
模块的方法:
import inspect
print(f"Line number in global scope: {inspect.currentframe().f_lineno}")
Other code
print(f"Line number in global scope: {inspect.currentframe().f_lineno}")
三、优化代码以便调试
在调试代码时,获取行号是非常有用的。通过结合使用 inspect
和 traceback
模块,可以轻松地跟踪代码执行的路径。例如:
import inspect
def debug_log(message):
frame = inspect.currentframe().f_back
line_number = frame.f_lineno
print(f"[DEBUG] {message} (Line {line_number})")
def some_function():
debug_log("Entered some_function")
# Other code
debug_log("Exiting some_function")
some_function()
在这个例子中,debug_log
函数会打印消息和行号,帮助开发者了解代码的执行路径。
四、在异常处理时获取行号
在处理异常时,获取行号也是非常重要的。例如:
import traceback
try:
raise ValueError("An error occurred")
except Exception as e:
stack = traceback.extract_stack()
_, _, _, line = stack[-2]
print(f"Exception occurred at line: {line}")
print(f"Error message: {e}")
在这个例子中,当异常发生时,traceback.extract_stack()
方法获取了堆栈信息,并打印了发生异常的行号和错误消息。
五、结合日志记录获取行号
在实际应用中,通常需要记录日志以便后续分析。可以结合 logging
模块和 inspect
模块来记录日志行号。例如:
import logging
import inspect
Configure logging
logging.basicConfig(level=logging.DEBUG, format='%(message)s')
def log_with_lineno(message):
frame = inspect.currentframe().f_back
line_number = frame.f_lineno
logging.debug(f"{message} (Line {line_number})")
def some_function():
log_with_lineno("Entered some_function")
# Other code
log_with_lineno("Exiting some_function")
some_function()
在这个例子中,log_with_lineno
函数会记录日志消息和行号,便于调试和分析。
六、在复杂项目中的应用
在大型复杂项目中,可能需要在多个文件和模块中打印行号。可以创建一个辅助模块来统一管理行号打印功能。例如:
# utils.py
import inspect
def print_line_number():
print(f"Current line number: {inspect.currentframe().f_back.f_lineno}")
main.py
from utils import print_line_number
def some_function():
print_line_number()
# Other code
print_line_number()
some_function()
通过这种方式,可以在项目的任何地方方便地打印行号,增强代码的可调试性。
七、使用装饰器自动打印行号
装饰器是一种非常强大的工具,可以用来自动打印函数调用时的行号。例如:
import inspect
def print_line_decorator(func):
def wrapper(*args, kwargs):
frame = inspect.currentframe().f_back
line_number = frame.f_lineno
print(f"Calling {func.__name__} at line {line_number}")
return func(*args, kwargs)
return wrapper
@print_line_decorator
def some_function():
print("Inside some_function")
some_function()
在这个例子中,print_line_decorator
装饰器会在调用 some_function
时自动打印调用行号。
八、结合调试器打印行号
在使用调试器(如 pdb
)时,可以结合打印行号来更好地理解代码执行。例如:
import pdb
import inspect
def some_function():
pdb.set_trace()
print(f"Line number inside function: {inspect.currentframe().f_lineno}")
# Other code
print(f"Line number inside function: {inspect.currentframe().f_lineno}")
some_function()
在这个例子中,pdb.set_trace()
会在执行到该行时暂停程序,并进入调试模式。可以使用 inspect
模块在调试模式下打印当前行号。
九、在单元测试中获取行号
在编写单元测试时,可以使用行号来帮助定位失败的测试用例。例如:
import unittest
import inspect
class TestExample(unittest.TestCase):
def test_something(self):
self.assertTrue(True)
print(f"Test line number: {inspect.currentframe().f_lineno}")
if __name__ == '__main__':
unittest.main()
在这个例子中,inspect.currentframe().f_lineno
会在测试用例中打印当前行号,便于调试和分析。
十、使用第三方库获取行号
有些第三方库可以提供更高级的功能来获取行号。例如,loguru
是一个流行的日志库,支持自动记录行号:
from loguru import logger
logger.add("file.log", format="{time} {level} {message} (Line {line})")
def some_function():
logger.info("Inside some_function")
# Other code
logger.info("Exiting some_function")
some_function()
在这个例子中,loguru
会自动记录日志消息和行号,提供更强大的日志记录功能。
十一、在多线程环境中获取行号
在多线程环境中获取行号可能会更加复杂,但仍然可以使用 inspect
模块。例如:
import threading
import inspect
def print_line_number():
print(f"Thread {threading.current_thread().name}: Line number {inspect.currentframe().f_back.f_lineno}")
def worker():
print_line_number()
# Other code
print_line_number()
threads = []
for i in range(5):
t = threading.Thread(target=worker, name=f"Thread-{i}")
threads.append(t)
t.start()
for t in threads:
t.join()
在这个例子中,每个线程会打印其当前行号,便于调试多线程代码。
十二、在异步编程中获取行号
在异步编程中获取行号也可以使用 inspect
模块。例如:
import asyncio
import inspect
async def print_line_number():
print(f"Line number: {inspect.currentframe().f_back.f_lineno}")
async def main():
await print_line_number()
# Other code
await print_line_number()
asyncio.run(main())
在这个例子中,异步函数 print_line_number
会打印当前行号,便于调试异步代码。
十三、在元编程中获取行号
在元编程中,动态生成代码时也可以获取行号。例如:
import inspect
def create_function():
def dynamic_function():
print(f"Line number in dynamic function: {inspect.currentframe().f_lineno}")
return dynamic_function
dynamic_func = create_function()
dynamic_func()
在这个例子中,动态生成的函数 dynamic_function
会打印其当前行号,便于调试生成的代码。
十四、总结
通过本文的介绍,可以看到在 Python 中获取和打印行号的方法有很多种。无论是在函数内部、类方法内部、全局范围、异常处理、日志记录、单元测试、第三方库、多线程环境、异步编程还是元编程中,都可以使用 inspect
模块或其他方法来获取行号。这些方法可以帮助开发者更好地理解代码的执行路径,增强代码的可调试性,提高代码质量和开发效率。
相关问答FAQs:
如何在Python中获取当前行号?
在Python中,可以使用inspect
模块来获取当前行号。通过调用inspect.currentframe()
获取当前帧,然后通过f_lineno
属性来获取行号。示例代码如下:
import inspect
def print_line_number():
frame = inspect.currentframe()
print(f"当前行号是: {frame.f_lineno}")
print_line_number()
有没有简单的方法在Python中输出行号?
如果您希望在代码中简单地打印行号,可以使用__line__
。在一些IDE中,可以通过print(__line__)
来输出当前行号,然而这不是Python语言的标准特性,主要依赖于开发环境。
如何在Python脚本中调试时显示行号?
使用调试工具如pdb
可以方便地查看代码的行号。在调试时,您可以设置断点并逐行执行代码,pdb
会显示当前行号和相关信息。可以通过在代码中插入import pdb; pdb.set_trace()
来启动调试模式。
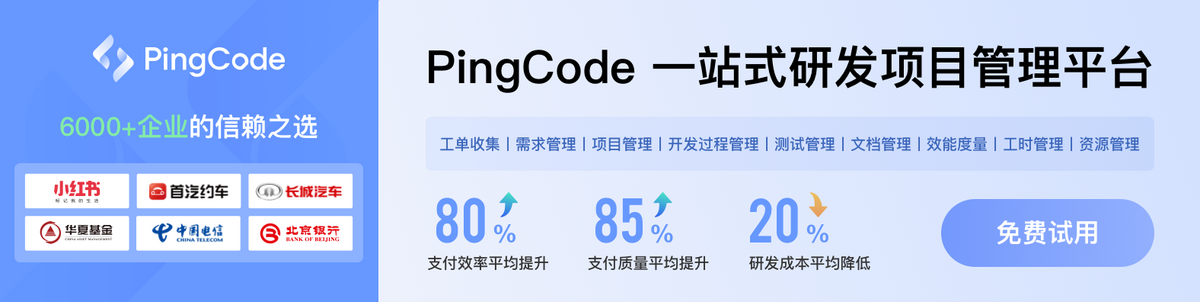