在Python中,建立字符串的方法包括使用单引号、双引号、三引号、字符串拼接、字符串格式化、字符串操作函数。使用单引号或双引号可以直接创建字符串,而三引号可以创建包含换行的多行字符串。字符串拼接可以通过加号操作符或join方法实现,字符串格式化可以使用format方法或f-string。下面将详细描述如何使用字符串格式化来建立字符串。
字符串格式化(f-string):
f-string是一种更简洁高效的字符串格式化方法,只需在字符串前加一个字母“f”或“F”,然后在字符串内使用花括号{}括起来的表达式即可。它不仅支持变量,还支持表达式和函数调用,且易于阅读和维护。
例如:
name = "Alice"
age = 30
使用f-string格式化字符串
formatted_string = f"Name: {name}, Age: {age}"
print(formatted_string)
输出结果为:
Name: Alice, Age: 30
一、字符串的基本创建方法
1、使用单引号和双引号
在Python中,可以使用单引号或双引号来创建字符串。这两种方法在功能上完全相同,主要区别在于当字符串本身包含单引号或双引号时选择使用另一种引号类型。
例如:
single_quote_str = 'Hello, World!'
double_quote_str = "Hello, World!"
当字符串中包含单引号时,可以使用双引号创建字符串,反之亦然:
str_with_single_quote = "It's a beautiful day!"
str_with_double_quote = 'He said, "Python is awesome!"'
2、使用三引号
三引号(单引号或双引号连续三次)用于创建包含换行符的多行字符串。这种方法非常适合定义文档字符串(docstring)或长文本。
例如:
multi_line_str = """This is a multi-line string.
It spans multiple lines.
This is useful for long text."""
print(multi_line_str)
输出结果为:
This is a multi-line string.
It spans multiple lines.
This is useful for long text.
二、字符串拼接方法
1、使用加号操作符
加号操作符(+)用于拼接两个或多个字符串。这种方法简单直观,但在拼接多个字符串时效率较低。
例如:
str1 = "Hello"
str2 = "World"
concatenated_str = str1 + ", " + str2 + "!"
print(concatenated_str)
输出结果为:
Hello, World!
2、使用join方法
join方法是推荐的字符串拼接方式,尤其在拼接多个字符串时效率更高。它通过将一个可迭代对象(如列表、元组)中的元素连接成一个字符串。
例如:
str_list = ["Hello", "World", "from", "Python"]
joined_str = " ".join(str_list)
print(joined_str)
输出结果为:
Hello World from Python
三、字符串格式化方法
1、使用%操作符
%操作符是一种传统的字符串格式化方法,类似于C语言中的printf。它通过在字符串中放置格式说明符,并在后面提供一个或多个值。
例如:
name = "Alice"
age = 30
formatted_str = "Name: %s, Age: %d" % (name, age)
print(formatted_str)
输出结果为:
Name: Alice, Age: 30
2、使用format方法
format方法通过在字符串中使用花括号{}作为占位符,并在后面调用format方法传递相应的值来实现字符串格式化。
例如:
name = "Alice"
age = 30
formatted_str = "Name: {}, Age: {}".format(name, age)
print(formatted_str)
输出结果为:
Name: Alice, Age: 30
四、字符串操作函数
1、字符串拆分
split方法用于将字符串拆分成一个列表。它通过指定的分隔符(默认为空格)将字符串拆分开。
例如:
sentence = "This is a sentence."
words = sentence.split()
print(words)
输出结果为:
['This', 'is', 'a', 'sentence.']
2、字符串替换
replace方法用于将字符串中的某个子字符串替换为另一个子字符串。
例如:
original_str = "Hello, World!"
new_str = original_str.replace("World", "Python")
print(new_str)
输出结果为:
Hello, Python!
3、字符串查找
find方法用于查找子字符串在字符串中的位置。如果找到,返回第一个匹配的位置索引,否则返回-1。
例如:
original_str = "Hello, World!"
position = original_str.find("World")
print(position)
输出结果为:
7
五、字符串常见操作及应用
1、字符串大小写转换
Python提供了多种方法来转换字符串的大小写,包括upper()、lower()、capitalize()、title()等。
例如:
original_str = "hello, world!"
upper_str = original_str.upper()
lower_str = original_str.lower()
capitalized_str = original_str.capitalize()
title_str = original_str.title()
print(upper_str) # HELLO, WORLD!
print(lower_str) # hello, world!
print(capitalized_str) # Hello, world!
print(title_str) # Hello, World!
2、移除字符串两端的空白字符
strip方法用于移除字符串两端的空白字符(包括空格、换行符等)。lstrip方法移除左端的空白字符,rstrip方法移除右端的空白字符。
例如:
original_str = " Hello, World! "
stripped_str = original_str.strip()
left_stripped_str = original_str.lstrip()
right_stripped_str = original_str.rstrip()
print(stripped_str) # "Hello, World!"
print(left_stripped_str) # "Hello, World! "
print(right_stripped_str) # " Hello, World!"
3、检查字符串是否以某个子字符串开头或结尾
startswith方法用于检查字符串是否以某个子字符串开头,endswith方法用于检查字符串是否以某个子字符串结尾。
例如:
original_str = "Hello, World!"
starts_with_hello = original_str.startswith("Hello")
ends_with_world = original_str.endswith("World!")
print(starts_with_hello) # True
print(ends_with_world) # True
六、字符串编码和解码
1、编码字符串
在Python中,字符串是Unicode字符的序列。通过encode方法可以将字符串编码为字节对象(bytes),常见的编码包括UTF-8、ASCII等。
例如:
original_str = "Hello, World!"
encoded_str = original_str.encode("utf-8")
print(encoded_str)
输出结果为:
b'Hello, World!'
2、解码字节对象
通过decode方法可以将字节对象解码为字符串。
例如:
encoded_str = b'Hello, World!'
decoded_str = encoded_str.decode("utf-8")
print(decoded_str)
输出结果为:
Hello, World!
七、字符串的切片操作
1、基本切片
切片操作允许从字符串中提取子字符串。切片操作的语法为[start:end:step],其中start是起始索引(包括),end是结束索引(不包括),step是步长(默认为1)。
例如:
original_str = "Hello, World!"
substring = original_str[0:5]
print(substring)
输出结果为:
Hello
2、步长切片
步长切片可以通过指定步长来提取字符串中的字符。
例如:
original_str = "Hello, World!"
substring = original_str[::2]
print(substring)
输出结果为:
Hlo ol!
八、字符串的常见内置方法
1、count方法
count方法用于统计子字符串在字符串中出现的次数。
例如:
original_str = "Hello, World! Hello, Python!"
count_hello = original_str.count("Hello")
print(count_hello)
输出结果为:
2
2、startswith方法和endswith方法
startswith方法用于检查字符串是否以某个子字符串开头,endswith方法用于检查字符串是否以某个子字符串结尾。
例如:
original_str = "Hello, World!"
starts_with_hello = original_str.startswith("Hello")
ends_with_world = original_str.endswith("World!")
print(starts_with_hello) # True
print(ends_with_world) # True
九、字符串的常见操作场景
1、处理用户输入
在用户输入时,通常需要对输入字符串进行处理,如去除两端空白字符、转换大小写、验证格式等。
例如:
user_input = input("Enter your name: ").strip().title()
print(f"Hello, {user_input}!")
2、生成动态内容
在生成动态内容时,字符串格式化非常有用。例如,在生成网页内容或日志记录时,可以使用格式化方法插入变量。
例如:
user_name = "Alice"
action = "logged in"
message = f"User {user_name} has {action}."
print(message)
输出结果为:
User Alice has logged in.
十、字符串的高级操作
1、正则表达式
正则表达式是处理字符串的强大工具,可以用于复杂的字符串匹配、替换等操作。在Python中,可以使用re模块。
例如:
import re
pattern = r'\d+'
original_str = "There are 123 apples and 456 oranges."
matches = re.findall(pattern, original_str)
print(matches)
输出结果为:
['123', '456']
2、字符串翻译
translate方法和maketrans方法用于字符替换。maketrans方法创建一个转换表,translate方法根据转换表替换字符串中的字符。
例如:
original_str = "hello, world!"
translation_table = str.maketrans("aeiou", "12345")
translated_str = original_str.translate(translation_table)
print(translated_str)
输出结果为:
h2ll4, w4rld!
十一、字符串的安全操作
1、避免字符串拼接中的代码注入
在处理用户输入时,使用字符串拼接可能导致代码注入攻击。应尽量使用参数化查询或其他安全方法。
例如,在SQL查询中:
# 不安全的做法(容易受到SQL注入攻击)
unsafe_query = "SELECT * FROM users WHERE username = '" + user_input + "'"
安全的做法(使用参数化查询)
cursor.execute("SELECT * FROM users WHERE username = %s", (user_input,))
2、验证和清理用户输入
在处理用户输入时,应验证输入的格式和内容,并进行必要的清理。
例如:
import re
user_input = input("Enter your email: ").strip()
if re.match(r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$', user_input):
print("Valid email address.")
else:
print("Invalid email address.")
十二、总结
在Python中,字符串操作是日常编程中最常见的任务之一。通过本文的介绍,您可以掌握如何创建字符串、拼接字符串、格式化字符串以及常见的字符串操作方法。无论是处理用户输入、生成动态内容,还是进行字符串匹配和替换,Python都提供了丰富的内置方法和工具。理解并熟练掌握这些方法,可以大大提高您的编程效率和代码质量。
相关问答FAQs:
如何在Python中创建多行字符串?
在Python中,可以使用三重引号('''或""")来创建多行字符串。这种方式允许在字符串中直接包含换行符。例如:
multiline_string = """这是第一行
这是第二行
这是第三行"""
这种方法非常适合编写长文本或文档字符串(docstring)。
Python中字符串的拼接方式有哪些?
字符串拼接可以通过多种方式实现。使用加号(+)运算符可以简单地将两个字符串连接在一起,例如:
string1 = "Hello, "
string2 = "World!"
result = string1 + string2
另外,使用join方法也是一种高效的拼接方式,尤其是在处理多个字符串时:
strings = ["Hello", "World"]
result = " ".join(strings)
在Python中如何对字符串进行格式化?
字符串格式化有几种常见的方法。使用f-string(格式化字符串字面量)是Python 3.6及以上版本的推荐方式,能够直接在字符串中插入变量:
name = "Alice"
greeting = f"Hello, {name}!"
另外,使用format()方法也很灵活:
greeting = "Hello, {}!".format(name)
通过这些方法,您可以轻松地构建动态字符串。
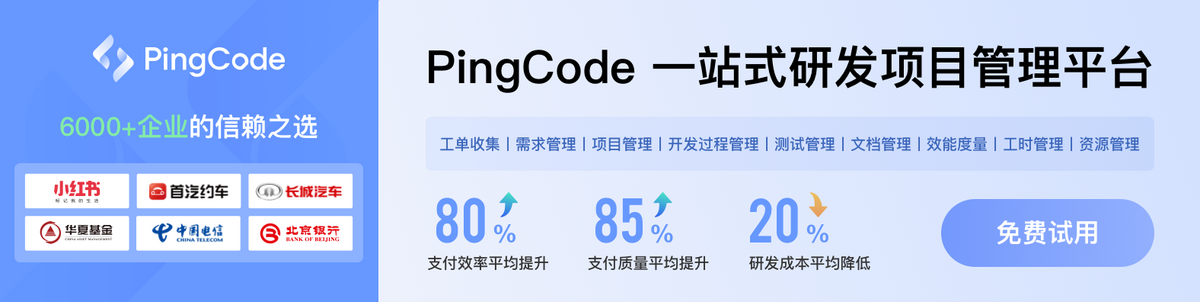