使用Python编程语言,我们可以通过多种方式来计算目录中的文件数量。常用的方法包括使用os模块、glob模块以及pathlib模块。这些方法各有优劣,适用于不同的使用场景。
一、使用os模块:
os模块是Python标准库的一部分,提供了与操作系统交互的功能。通过os模块,我们可以列出目录中的所有文件,并统计文件数量。
import os
def count_files_with_os(directory):
count = 0
for root, dirs, files in os.walk(directory):
count += len(files)
return count
directory_path = '/path/to/directory'
print(f'Total number of files: {count_files_with_os(directory_path)}')
上述代码中,os.walk
函数会递归遍历目录,并返回一个生成器。每次迭代会返回一个三元组(root, dirs, files),其中root是当前目录路径,dirs是目录列表,files是文件列表。通过累加文件列表的长度即可得到文件总数。
二、使用glob模块:
glob模块提供了文件名模式匹配功能,可以使用通配符来匹配文件和目录。
import glob
def count_files_with_glob(directory):
files = glob.glob(f'{directory}//*', recursive=True)
return len([file for file in files if os.path.isfile(file)])
directory_path = '/path/to/directory'
print(f'Total number of files: {count_files_with_glob(directory_path)}')
在上述代码中,glob.glob
函数使用递归方式匹配目录下的所有文件和子目录,返回匹配的路径列表。然后通过列表推导式过滤出文件路径,并统计文件数量。
三、使用pathlib模块:
pathlib模块是Python 3.4引入的面向对象的路径操作库,提供了更加直观和简洁的路径操作方法。
from pathlib import Path
def count_files_with_pathlib(directory):
return sum(1 for _ in Path(directory).rglob('*') if _.is_file())
directory_path = '/path/to/directory'
print(f'Total number of files: {count_files_with_pathlib(directory_path)}')
上述代码中,Path(directory).rglob('*')
方法会递归遍历目录,并返回一个生成器。通过生成器表达式过滤出文件路径,并统计文件数量。
四、性能比较:
不同方法在处理大目录时的性能可能有所不同。一般来说,使用os模块的性能会比较优越,因为它直接调用了底层操作系统的API。glob模块和pathlib模块的性能可能稍逊一筹,但它们提供了更高层次的抽象和更简洁的代码。
为了更好地理解这些方法的性能,可以编写测试代码对比它们在处理大目录时的执行时间:
import time
directory_path = '/path/to/large/directory'
start = time.time()
count_files_with_os(directory_path)
print(f'os module: {time.time() - start:.4f} seconds')
start = time.time()
count_files_with_glob(directory_path)
print(f'glob module: {time.time() - start:.4f} seconds')
start = time.time()
count_files_with_pathlib(directory_path)
print(f'pathlib module: {time.time() - start:.4f} seconds')
五、错误处理:
在实际应用中,目录可能不存在或权限不足。在这些情况下,需要处理可能发生的异常。
import os
from pathlib import Path
import glob
def count_files_with_error_handling(directory):
try:
count = 0
for root, dirs, files in os.walk(directory):
count += len(files)
return count
except Exception as e:
print(f'Error: {e}')
return 0
def count_files_with_glob_error_handling(directory):
try:
files = glob.glob(f'{directory}//*', recursive=True)
return len([file for file in files if os.path.isfile(file)])
except Exception as e:
print(f'Error: {e}')
return 0
def count_files_with_pathlib_error_handling(directory):
try:
return sum(1 for _ in Path(directory).rglob('*') if _.is_file())
except Exception as e:
print(f'Error: {e}')
return 0
directory_path = '/path/to/directory'
print(f'Total number of files: {count_files_with_error_handling(directory_path)}')
print(f'Total number of files: {count_files_with_glob_error_handling(directory_path)}')
print(f'Total number of files: {count_files_with_pathlib_error_handling(directory_path)}')
在上述代码中,通过try-except语句捕获可能的异常,并在发生异常时打印错误信息并返回0。这样可以确保程序在异常情况下不会崩溃,并提供有用的调试信息。
六、总结:
Python提供了多种方法来统计目录中的文件数量,常用的方法包括os模块、glob模块和pathlib模块。每种方法都有其优缺点,适用于不同的使用场景。在实际应用中,可以根据需求选择合适的方法,并在处理大目录时注意性能问题。此外,还需要考虑可能的异常情况,并进行适当的错误处理。
通过本文的介绍,相信读者已经掌握了如何使用Python统计目录中的文件数量,并了解了不同方法的实现细节和性能对比。希望这些知识能够在实际开发中为读者提供帮助,提高代码的健壮性和可维护性。
相关问答FAQs:
如何在Python中读取文件中的数字?
在Python中,可以使用内置的文件操作功能来读取文件中的内容。通过逐行读取文件,可以使用正则表达式或字符串方法提取数字。示例代码如下:
import re
with open('your_file.txt', 'r') as file:
content = file.read()
numbers = re.findall(r'\d+', content)
print(numbers)
这段代码会提取并打印出文件中所有的数字。
如何计算文件中数字的总和?
要计算文件中所有数字的总和,可以在读取并提取数字后,将其转换为整数并进行累加。以下是实现的示例:
total_sum = sum(int(num) for num in numbers)
print("文件中数字的总和为:", total_sum)
结合前面的代码,这样可以轻松获取文件中所有数字的总和。
如何处理文件中不规则的数字格式?
如果文件中包含不规则的数字格式,比如带有符号的数字(如负数)或小数点,可以调整正则表达式以匹配这些情况。以下是一个示例:
numbers = re.findall(r'-?\d+\.?\d*', content)
这个正则表达式可以提取出正数和负数,以及整数和浮点数。根据需要,可以进一步处理这些提取出的数字。
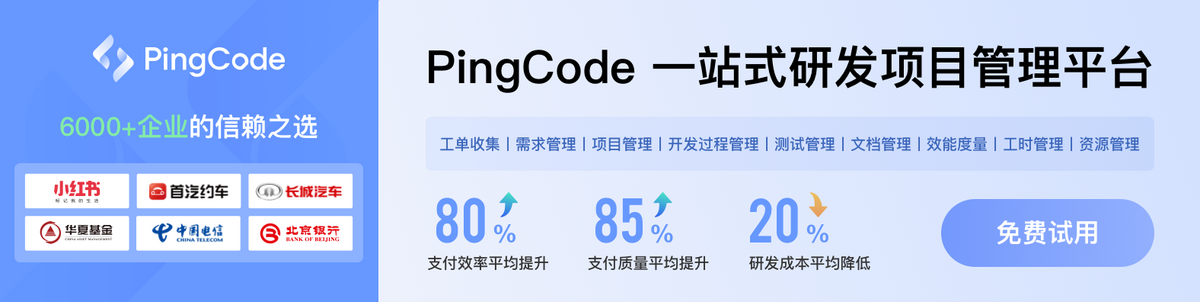